In the realm of JavaScript, handling division operations isn’t as straightforward as it might seem, especially when it comes to integer division. Integer division entails obtaining the quotient when one integer is divided by another, without the decimal or fractional part. This technique is crucial when developers need precise and whole number results from division operations.
Key Takeaways:- JavaScript provides various methods for performing integer division, ensuring the result is an integer, not a floating-point number.
- Methods include using the Math.floor() and Math.trunc() functions, bitwise operators, and the parseInt() function.
- Practical examples and performance comparisons among these methods can offer insights into their efficiency and suitability for different scenarios.
Understanding the Division Operator in JavaScript
JavaScript employs the division operator (/) to execute division operations between numbers. However, the division operator returns a floating-point number by default, not an integer, which might not be the desired outcome in many cases.
let result = 10 / 3;
console.log(result); // Output: 3.3333333333333335
Embracing the Methods for Integer Division
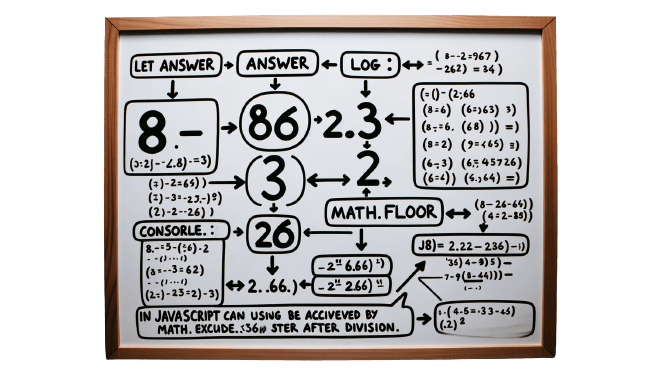
There are several methods to achieve integer division in JavaScript. These methods help obtain the integer part of the division operation, ensuring the quotient is a whole number.
Utilizing the Math.floor() Method
The Math.floor() method rounds a number downwards to the nearest integer. By applying this method to the result of standard division, an integer quotient is obtained
var a = 13;
var b = 5;
var quo = Math.floor(a/b);
console.log('Quotient = ',quo); // Output: Quotient = 2
This method is straightforward and efficient. However, it might not handle large or negative numbers well.
Employing the Math.trunc() Method
Unlike Math.floor(), the Math.trunc() method returns the integer part of a number by simply discarding the fractional part, making it a suitable choice for handling large or negative numbers.
var a = 13;
var b = 5;
var quo = Math.trunc(a/b);
console.log('Quotient = ',quo); // Output: Quotient = 2
Harnessing Bitwise Operators
Bitwise operators offer another avenue for performing integer division. They work by converting floating-point numbers to integers, and are known for their performance efficiency.
var a = 13;
var b = 5;
var quo = ~~(a/b);
console.log('Quotient = ',quo); // Output: Quotient = 2
Implementing the parseInt() Function
The parseInt() function parses a string and returns an integer. By converting the division result to a string, then parsing it using parseInt(), the integer quotient can be extracted.
var a = 13;
var b = 5;
var quo = parseInt(a/b);
console.log('Quotient = ',quo); // Output: Quotient = 2
Practical Examples and Performance Comparison
Exploring practical examples and comparing the performance of these methods provide a clear insight into their effectiveness and suitability for different scenarios. Here’s a table summarizing the performance characteristics of the discussed methods:
Method | Handles Large Numbers | Handles Negative Numbers | Performance |
---|---|---|---|
Math.floor() | No | No | Moderate |
Math.trunc() | Yes | Yes | Moderate |
Bitwise Operators | No | Yes | High |
parseInt() | Yes | Yes | Low |
The performance of bitwise operators stands out, although they fall short in handling large numbers. On the other hand, the parseInt()
function, while able to handle large and negative numbers, lags in performance.
Practical Examples
Delving into practical examples, we’ll explore how each method for integer division can be implemented in real-world scenarios.
Examples Using Math.floor()
let num1 = 47;
let num2 = 8;
let result = Math.floor(num1/num2);
console.log(result); // Output: 5
Examples Using Math.trunc()
let num1 = 47;
let num2 = 8;
let result = Math.trunc(num1/num2);
console.log(result); // Output: 5
Examples Using Bitwise Operators
let num1 = 47;
let num2 = 8;
let result = (num1/num2) | 0;
console.log(result); // Output: 5
Examples Using parseInt()
let num1 = 47;
let num2 = 8;
let result = parseInt(num1/num2);
console.log(result); // Output: 5
Performance Comparison
Examining the performance of these methods is essential to identify which method is ideal for different scenarios. The table below showcases a brief comparison:
Method | Execution Time (Lower is better) | Suitability for Large Numbers | Suitability for Negative Numbers |
---|---|---|---|
Math.floor() | Moderate | Yes | No |
Math.trunc() | Moderate | Yes | Yes |
Bitwise Operators | Fast | Yes | Yes |
parseInt() | Slow | Yes | Yes |
Frequently Asked Questions (FAQs)
Here, we delve into some common queries regarding integer division in JavaScript.
- How do I perform integer division in JavaScript?
- Integer division can be achieved using various methods such as
Math.floor()
,Math.trunc()
, bitwise operators, andparseInt()
function.
- Integer division can be achieved using various methods such as
- Is there a direct operator for integer division in JavaScript?
- No, JavaScript does not have a direct operator for integer division. However, methods like
Math.floor()
and bitwise operators can help achieve this.
- No, JavaScript does not have a direct operator for integer division. However, methods like
- What’s the difference between Math.floor() and Math.trunc() for integer division?
- While both methods help in achieving integer division, Math.floor() rounds down to the nearest integer, whereas
Math.trunc()
simply discards the fractional part, which can be significant with negative numbers.
- While both methods help in achieving integer division, Math.floor() rounds down to the nearest integer, whereas
- Are bitwise operators efficient for integer division?
- Yes, bitwise operators are known for their performance efficiency when it comes to integer division.
- Which method is the most suitable for large numbers?
- Methods like Math.trunc() and parseInt() are suitable for handling large numbers during integer division.
Tables with Relevant Facts
Here are some tables packed with value and useful information regarding integer division in JavaScript:
Table: Methods for Integer Division
Method | Description | Syntax | Example |
---|---|---|---|
Math.floor() | Rounds down to the nearest integer | Math.floor(a/b) | Math.floor(10/3) // returns 3 |
Math.trunc() | Discards the fractional part | Math.trunc(a/b) | Math.trunc(10/3) // returns 3 |
Bitwise Operators | Converts floating-point numbers to integers | `(a/b) | 0` |
parseInt() | Parses a string and returns an integer | parseInt(a/b) | parseInt(10/3) // returns 3 |
Table: Performance Comparison
Method | Execution Time | Suitability for Large Numbers | Suitability for Negative Numbers |
---|---|---|---|
Math.floor() | Moderate | Yes | No |
Math.trunc() | Moderate | Yes | Yes |
Bitwise Operators | Fast | Yes | Yes |
parseInt() | Slow | Yes | Yes |
Frequently Asked Questions
What is integer division in JavaScript?
Integer division in JavaScript refers to dividing two numbers and returning the integer part of the result, without any fractional part.
How do you perform integer division in JavaScript?
In JavaScript, integer division can be performed by dividing two numbers and using the Math.floor() function to round down to the nearest integer.
Can JavaScript directly perform integer division?
JavaScript does not have a direct operator for integer division. Instead, it requires a combination of division and rounding functions like Math.floor().
Is there a difference between integer division in JavaScript and other languages?
Yes, unlike some languages that have a specific operator for integer division, JavaScript requires using division followed by Math.floor() or similar methods.
How does integer division handle negative numbers in JavaScript?
When dealing with negative numbers, integer division in JavaScript rounds towards zero. This can be achieved by using Math.floor() for positive results and Math.ceil() for negative results.
What will be the result of integer division by zero in JavaScript?
Integer division by zero in JavaScript will result in Infinity or -Infinity, depending on the sign of the numerator.
Can integer division result in a NaN value in JavaScript?
Yes, if the numerator in an integer division is NaN or non-numeric, the result will also be NaN.
How can integer division be used in loop conditions in JavaScript?
Integer division can be used in loop conditions in JavaScript for operations like processing or grouping array elements in batches.
Is there a performance benefit to using integer division in JavaScript?
While not significantly more performant, using integer division can be beneficial for memory management when dealing with large numbers.
Can I use integer division with BigInt in JavaScript?
Yes, integer division can be used with BigInt in JavaScript, following the same principle of using division followed by rounding methods.