Are you interested in exploring Palindrome in Java? Let’s delve into the details in this comprehensive guide.
Introduction to Palindrome In Java
Palindromes, fascinating sequences of characters, have the unique property of reading the same forwards and backwards.
Now, let’s explore some captivating examples of palindrome patterns.
Numeric Palindromes
Examples of Palindrome Numbers: 11, 121, 333, 5555 Palindromic Time: 12:21, 13:31, 11:11Palindrome Words, Sentences, and Names
1. Palindromic Words
Words like “rotor,” “civic,” “level,” and “racecar.”
2. Palindromic Names
Names such as “anna,” “eve,” and “bob.”
3. Palindromic Sentences
Sentences like “Was it a car or a cat I saw,” “Mr. Owl ate my metal worm,” and “Do geese see god.”
Using Java for Palindrome Validation
Let’s embark on a journey to create a Java program that can determine whether a given number is a palindrome.
In our example, we’ll use the number 151, declared as an integer variable.
To achieve this, we’ll employ the logic of a for loop for iteration.
Furthermore, we’ll introduce a temporary variable called “actualValue” to store the original string and perform a comparison with its reversed counterpart.
Palindrome Number Validation Using a For Loop
public static void main(String[] args) {
try {
int number = 151;
int reverseValue = 0;
int reminder;
int actualValue;
actualValue = number;
for(;number!= 0; number /= 10) {
reminder = number % 10;
reverseValue = reverseValue * 10 + reminder;
}
if (actualValue == reverseValue)
System.out.println(actualValue + " is a valid palindrome.");
else
System.out.println(actualValue + " is not a valid palindrome.");
} catch (Exception e) {
e.printStackTrace();
}
}
Output:
151 is a valid palindrome.
As demonstrated by the above output, the original input number, 151, matches its reversed form, resulting in a valid palindrome.
Now, let’s explore an alternative approach using a while loop in the Java program below.
public static void main(String[] args) {
try {
int number = 151;
int reverseValue = 0;
int reminder;
int actualValue;
actualValue = number;
while (number != 0) {
reminder = number % 10;
reverseValue = reverseValue * 10 + reminder;
number /= 10;
}
if (actualValue == reverseValue)
System.out.println(actualValue + " is a valid palindrome.");
else
System.out.println(actualValue + " is not a valid palindrome.");
} catch (Exception e) {
e.printStackTrace();
}
}
Output:
151 is a valid palindrome.
As seen in the above output, the original number matches its reversed form.
In essence, a palindrome reads the same from left to right and right to left, much like our number 151.
You May Also Be Interested In:
- How to Remove a Character from a String in Python
- 4 Ways to Remove Characters from a String in JavaScript
Validating Palindrome Strings
In this section, we’ll explore the validation of strings as palindromes in Java.
Let’s create a Palindrome program to determine whether an input string is a palindrome or not.
Here’s an example:
public static void main(String[] args) {
try {
String inputValue = "rotor";
String reverseValue = "";
int length = inputValue.length();
for (int x = length - 1; x >= 0; x--)
reverseValue = reverseValue + inputValue.charAt(x);
if (inputValue.equals(reverseValue))
System.out.println(inputValue + " is a valid palindrome.");
else
System.out.println(inputValue + " is not a valid palindrome.");
} catch (Exception e) {
e.printStackTrace();
}
}
Output:
rotor is a valid palindrome.
As demonstrated by the above output, the input character sequence “rotor” matches its reversed form, resulting in a valid palindrome.
Palindrome Validation Using the Reverse Method
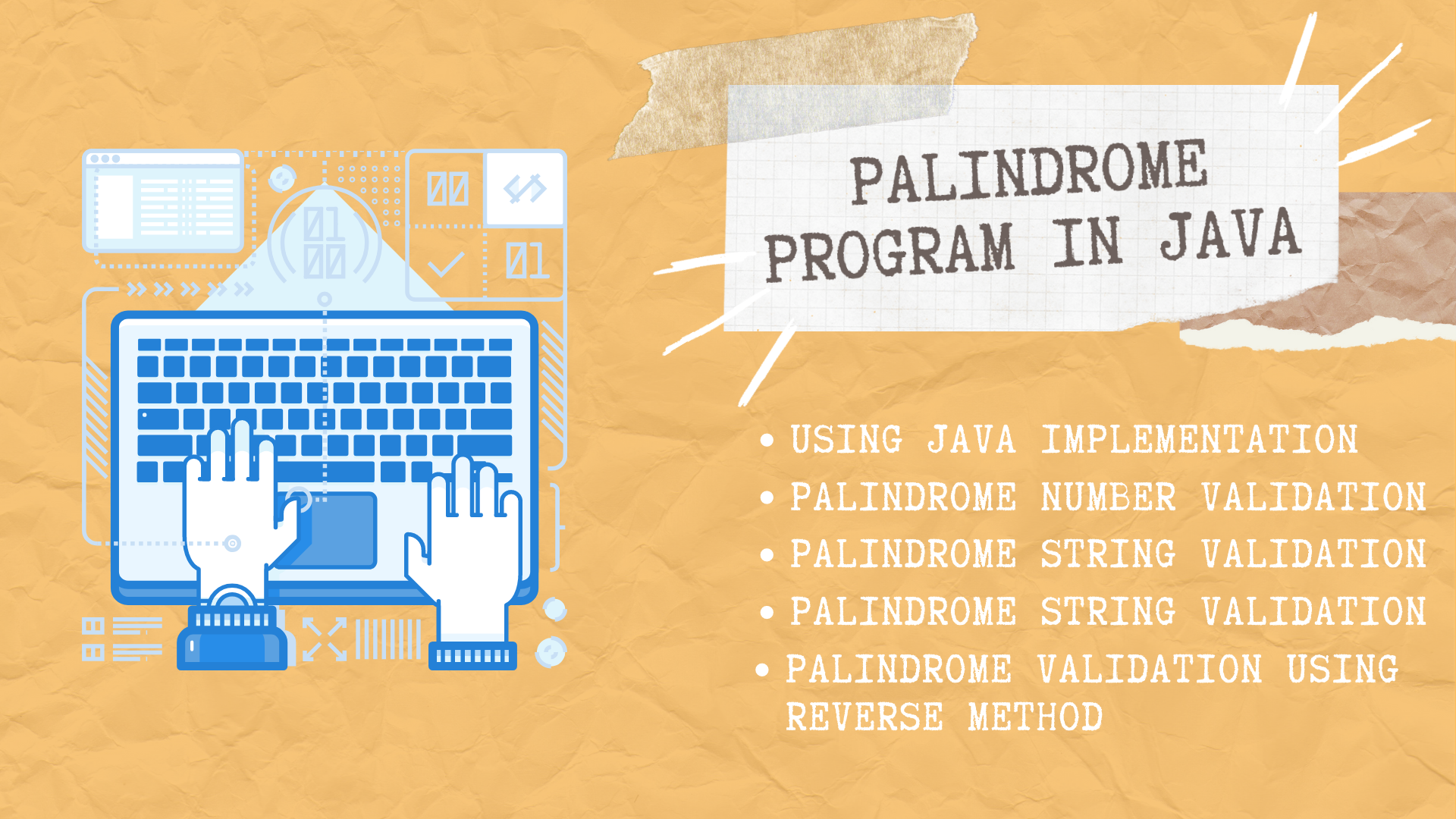
In this section, we’ll explore palindrome validation in Java using the reverse method.
We’ll make use of the `reverse()` method from the Java library’s StringBuffer.
With the following Palindrome program, we’ll check if a given string is a palindrome.
The program will compare the original string with its reversed version and provide the result accordingly.
public static void main(String[] args) {
try {
String inputValue ="Ana";
String reverseValue = new StringBuffer(inputValue).reverse().toString();
if (inputValue.equalsIgnoreCase(reverseValue))
System.out.println(inputValue + " is a valid palindrome.");
else
System.out.println(inputValue + " is not a valid palindrome.");
} catch (Exception e) {
e.printStackTrace();
}
}
Output:
Ana is a valid palindrome.
As evident from the output above, the input “Ana” matches its reversed form, resulting in a valid palindrome.
In conclusion, this tutorial has covered various methods for validating palindromes in Java.
Frequently Asked Questions (FAQs)
What is a palindrome in Java?
A palindrome in Java is a sequence of characters that reads the same forwards and backwards, ignoring spaces, punctuation, and capitalization.
How can I check if a string is a palindrome in Java?
You can check if a string is a palindrome in Java by comparing the original string with its reverse. If they are the same, the string is a palindrome.
What is the most efficient way to check for a palindrome in Java?
The most efficient way to check for a palindrome in Java is to iterate through the string from both ends towards the center, comparing characters as you go.
Can palindromes contain special characters or spaces?
Yes, palindromes in Java can contain special characters and spaces, but these should be ignored when checking for palindromic properties.
Are palindromes case-sensitive in Java?
No, palindromes in Java are typically not case-sensitive. Uppercase and lowercase characters are considered the same when checking for palindromes.
What are some examples of palindromic strings in Java?
Examples of palindromic strings in Java include ‘racecar’, ‘madam’, and ‘A man, a plan, a canal, Panama!’
How can I handle spaces and special characters when checking for palindromes?
To handle spaces and special characters, you can preprocess the string by removing or ignoring them before checking for palindromes.
Is there a built-in method in Java for checking palindromes?
Java does not have a built-in method specifically for checking palindromes, but you can write a custom function to perform the check.
What is the time complexity of checking a palindrome in Java?
The time complexity of checking a palindrome in Java is typically O(n), where n is the length of the string.
Can palindromes be numeric in Java?
Yes, palindromes in Java can be numeric, containing numbers that read the same forwards and backwards.