Print Exception Python: In any programming environment, errors are inevitable ghosts in the code that developers must chase. Python, like other languages, has a robust system for handling them, making it easier to deal with the myriad of errors that could occur. This python tutorial dives into the core concepts of Python exceptions, explores ways to handle them effectively, and equips you with the tools to build reliable and resilient programs.
Key Takeaways:- Grasping the core concepts of Python exceptions and their significance.
- Familiarity with Python basics around common exceptions encountered.
- Understanding the syntax and utilization of the Try Except block.
- Exploring built-in python exception classes and their hierarchy in Python.
- Mastering various techniques effectively, including printing exceptions and accessing tracebacks.
Understanding Python Exceptions
Introduction to Python Exceptions
Imagine a scenario where you expect a file to be present, but it’s missing. This unexpected event triggers a FileNotFoundError
, notifying you of the discrepancy. Exceptions act as real-world standard error analogies in your code, allowing you to handle them gracefully and prevent python program crashes.
Defining the Terms:
- Error: A problem in the code that halts program execution.
- Exception: A special type of error that can be caught and handled.
- Traceback: A traceback module is a detailed record of the sequence of function calls that led to an exception, providing valuable insights for debugging.
Built-in Exception Classes:
All exceptions in Python belong to classes derived from the base Exception class. This hierarchy facilitates structured handling of different groups of exceptions.
Here’s a glimpse of the hierarchy:
- Base Class: Exception
- Derived Classes: ArithmeticError, LookupError, EnvironmentError, etc.
Commonly Used Built-in Classes:
- OSError: Base class for I/O-related errors.
- IndexError: Raised when a sequence subscript is out of range.
- ZeroDivisionError: Raised when attempting to divide by zero.
Real-world Analogy
try:
with open('file.txt', 'r') as file:
content = file.read()
except FileNotFoundError as e:
print(f"Error: {e}")
In the above code snippet, if file.txt
doesn’t exist, Python will throw a FileNotFoundError
. However, due to the try-except
block, this error will be caught and a user-friendly message will be printed instead of halting the program.
Common Types of Exceptions in Python
This infographic summarizes common exception types in Python.
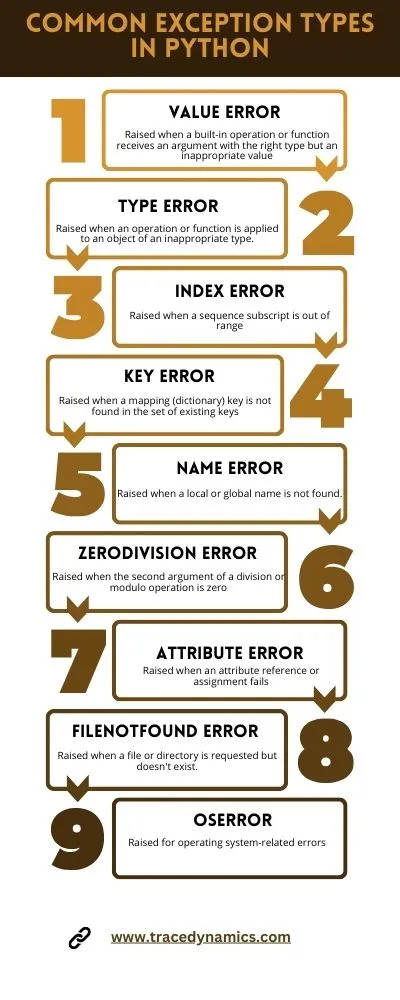
Python has a number of built-in exceptions that are raised when your program encounters an error (something in the program goes awry). Here are some common mutiple exception you might encounter:
SyntaxError
: Raised when there is an error in Python syntax.TypeError
: Raised when an operation or function is applied to an object of inappropriate type.ValueError
: Raised when a built-in operation or function receives an argument that has the right type but an inappropriate value.
Unraveling Specific Exceptions: A Closer Look
While Python’s built-in exceptions cover a wide range of errors, let’s dive deeper into a few common ones to uncover their causes, consequences, and best practices for handling them gracefully:
KeyError: When Dictionaries Get Disgruntled
Imagine a dictionary as a meticulous librarian who demands precision when accessing its books (values). If you request a book by a title (key) that doesn’t exist, they’ll throw a KeyError, exclaiming, “That book isn’t in our collection!”
Common scenarios:
Searching for a non-existent key in a dictionary:
my_dict = {"name": "Alice", "age": 30}
try:
print(my_dict["occupation"]) # KeyError: 'occupation'
except KeyError as e:
print("Oops, missing key:", e)
Handling user input to access dictionary values:
user_choice = input("Choose a fruit: apple, banana, or orange: ")
try:
print("Your choice:", fruits[user_choice]) # Potential KeyError
except KeyError:
print("Invalid fruit choice. Please try again.")
Best practices:
Use get() for key retrieval with a default value:
occupation = my_dict.get("occupation", "Not specified")
Check for key existence with in before accessing:
if "occupation" in my_dict:
print(my_dict["occupation"])
IndexError: Out of Bounds with Lists and Tuples
Lists and tuples behave like organized shelves, each item indexed by a number. But venturing beyond their boundaries triggers an IndexError, a stern reminder that you’re trying to access an item that doesn’t exist.
Common scenarios:
Accessing elements beyond list or tuple length:
my_list = [10, 20, 30]
try:
print(my_list[3]) # IndexError: list index out of range
except IndexError:
print("Index out of bounds!")
Using negative indices incorrectly:
my_tuple = (5, 10)
try:
print(my_tuple[-3]) # IndexError: tuple index out of range
except IndexError:
print("Invalid negative index.")
Best practices:
- Double-check list or tuple lengths before indexing.
- Use positive indices starting from 0.
- Be mindful of negative indices, which count from the end.
Stay tuned as we delve into more intriguing exception types, unraveling their mysteries and unlocking best practices for handling them like a Python pro!
The Try-Except Block
block forms the bedrock of exception handling in Python. The try clause block houses code that might raise an specific exception, while the except
block defines how to handle it.
try:
# code that may raise exception
num = int("string")
except ValueError as ve:
print(f"Error: {ve}")
In the code above, a ValueError
will be raised because you cannot convert a string to an integer. The try-except
block catches the error and prints a user-friendly message.
Syntax and Usage
- The
try
block: Contains code that may raise an exception. - The
except
block: Contains code that will execute if an exception occurs.
Example Scenarios
- Reading a file that may not exist.
- User input validation.
The below flowchart illustrates the try, catch and finally clause:
Handling Exceptions in Python
Handling or raising exceptions effectively is crucial for building robust applications. In Python, exception handling encompasses a broad spectrum from printing exception to logging them for post-mortem analysis. This section delves into various techniques to handle exceptions in Python
Printing Exceptions
Printing exceptions is a fundamental technique that provides valuable insights into the error without crashing the program. You can use the print
function to display the exception message and type for better understanding.
Using the print
Function to Display Exceptions
try:
# code that may raise exception
num = int("string")
except ValueError as ve:
print(f"Error: {ve}")
In this code, if a ValueError
occurs, its message and type will be printed, providing helpful information for debugging.
Formatting Exceptions for Better Readability
Python provides various ways to format exceptions, making it easier to understand the error.
try:
# code that may raise exception
num = int("string")
except ValueError as ve:
print(f"Error: {ve}")
print(f"Exception Type: {type(ve)}")
print(f"Traceback: {traceback.print_exc()}") # Prints the traceback
In this code, if a ValueError occurs, the message, type, and traceback will be printed, providing a comprehensive understanding of the error’s context.
Understanding the Power of the Python Traceback:
While we’ve been discussing how to catch and handle exceptions in Python, there’s another crucial tool in our debugging arsenal: the traceback. Think of it as a detective’s roadmap, leading you step-by-step through the code that led to the error. Understanding and analyzing tracebacks effectively can dramatically improve your debugging skills and help you pinpoint the culprit quickly.
What is a Traceback?
Imagine you’re making a delicious casserole, but it ends up tasting slightly off. Tracing back your steps helps you identify the ingredient or step that went wrong. Similarly, a traceback is a record of the function calls leading up to the error, offering a detailed chronological narrative of what went wrong.
Here’s an example:
def calculate_discount(price, discount_rate):
try:
final_price = price * (1 - discount_rate)
return final_price
except TypeError:
print("Error: Invalid input types!")
original_price = "100"
discount = 0.5
try:
final_price = calculate_discount(original_price, discount)
print(f"Final price: ${final_price}")
except TypeError:
print("Please enter valid number values.")
Running this code would result in a TypeError because “100” is a string, not a number. The traceback would look something like this:
Traceback (most recent call last):
File "recipe.py", line 6, in
final_price = calculate_discount(original_price, discount)
File "recipe.py", line 2, in calculate_discount
final_price = price * (1 - discount_rate)
TypeError: unsupported operand type(s) for *: 'str' and 'float'
This traceback tells us:
- The error occurred on line 2 of calculate_discount.
- It’s a TypeError because of an unsupported operation between a string and a float.
- The call originated from line 6 in the main block.
Decoding the Traceback:
Each line in the traceback represents a function call, with the most recent call at the bottom. Reading from the bottom up, you can trace the path the program took before encountering the error.
Analyzing the elements helps you pinpoint the issue:
- Filename and line number: Identify the exact location of the error.
- Function names: Understand the sequence of function calls leading to the error.
- Error message: Get an immediate clue about the nature of the issue.
Think of the traceback as a series of breadcrumbs leading you directly to the source of the problem. Analyzing each step systematically will help you fix the bug and prevent similar issues in the future.
Mastering Traceback Analysis:
Here are some additional tips for effectively using tracebacks:
- Shorten tracebacks: Use the traceback.format_exc() function to shorten long tracebacks for easier reading.
- Visualize the stack: Utilize tools like debuggers or online visualizers to create interactive stack diagrams for clearer understanding.
- Leverage additional resources: Consult online documentation and tutorials to learn about advanced traceback analysis techniques.
By incorporating these practices, you can transform from a passive traceback observer to a skilled code detective, confidently navigating the error landscape and ultimately delivering robust and well-functioning Python applications.
Remember: The next time you encounter an error in your Python code, don’t be intimidated by the traceback. Embrace it as a valuable tool to uncover the problem and write flawless code!
User-Friendly Exceptions: Turning Cryptic Errors into Helpful Guides
Errors are inevitable, but cryptic technical jargon shouldn’t be the norm. In Python, user-friendly exception printing transforms confusing messages into valuable tools that guide users towards solutions. Let’s explore best practices for making your exceptions helpful and informative:
- Ditch the Jargon: Translate technical terms like “ValueError” or “IndexError” into plain English. Think of explaining the error to a friend unfamiliar with coding. For example, instead of “TypeError: unsupported operand type(s) for +: ‘str’ and ‘int’,” say, “Oops! Adding text and numbers isn’t allowed here. Please use only numbers for this calculation.”
- Context is Key: Don’t leave users lost in the code. Provide context around the error by mentioning the specific function or line where it occurred. This saves them time hunting for the culprit. For example, “An error occurred while calculating your discount in the checkout function. Please double-check your discount code.”
- Offer a Helping Hand: Don’t just point out the problem; offer solutions! Suggest what the user can do to fix the error. For example, “Looks like you entered an invalid date format. Please use YYYY-MM-DD format for the date field.”
- Prioritize Like a Pro: Not all errors are created equal. Use different logging levels to distinguish minor hiccups from major roadblocks. A missing comma shouldn’t trigger panic, while a server crash deserves a bold “ERROR” flag.
- Format for Clarity: A wall of text is nobody’s friend. Use formatting like bullet points, indentation, or even colored text to make your error messages easy to scan and understand.
- Think Globally, Act Locally: Is your application reaching a global audience? Consider localization! Translate your error messages into different languages to ensure all users receive clear guidance regardless of their native tongue.
- Learn from the Best: Utilize libraries like prettyprinter or colorama to leverage their expertise in formatting and coloring error messages for optimal user experience.
- Less is More: While context and suggestions are valuable, avoid overwhelming users. Keep your messages concise and actionable. Remember, clarity and brevity are key to effective communication.
- Test and Iterate: Don’t assume your first draft is perfect. Test your user-friendly error messages with real users and gather their feedback. Use their insights to refine your messages for maximum impact.
- Remember the Mission: The ultimate goal is to empower users to navigate errors without technical expertise. By following these tips and prioritizing user-friendliness, you can turn frustrating roadblocks into manageable bumps on their code journey.
Logging Exceptions
Logging exceptions is a step beyond merely printing them. It records exceptions in a log file, aiding in debugging post-mortem.
Introduction to Logging in Python
Python’s logging module provides a flexible framework for emitting log messages from Python programs. It’s part of the Python Standard Library, so you don’t need to install anything to use it.
import logging
try:
# code that may raise exception
num = int("string")
except ValueError as ve:
logging.exception("Error")
In this code snippet, the logging.exception
method logs the exception with a message “Error”.
How to Log Exceptions
Logging exceptions involve creating a logging configuration and using logging methods to record exceptions.
import logging
logging.basicConfig(filename='example.log', level=logging.ERROR)
try:
# code that may raise exception
num = int("string")
except ValueError as ve:
logging.exception("Error")
In this code snippet, the logging.basicConfig
method is used to configure the logging, and logging.exception
is used to log the exception.
Custom Exception Classes
Creating user-defined exceptions allows for creating self-explanatory error messages and handling exceptions at a granular level.
Creating User-Defined Exceptions
class CustomError(Exception):
pass
try:
raise CustomError("A custom error occurred")
except CustomError as ce:
print(ce)
In this code snippet, a custom exception class CustomError
is created, which is derived from the base Exception
class.
Use Cases for Custom Exceptions
Custom exceptions can be used to create program-specific error conditions. They can also be used to create a hierarchy of exception classes for a large codebase.
Advanced Techniques:
Advanced exception handling involves techniques that provide more control over the exception handling/error handling process.
- Logging: Record exception name in a log file for post-mortem analysis.
- Custom Exceptions: Create custom exception classes for program-specific error conditions.
- Exception Chaining: Handle chained exceptions when one exception occurs while handling another.
- Context Managers: Simplify resource management and exception handling using
with
statements.
Exception Chaining
Exception chaining is used when an exception occurs while handling another exception.
try:
# code that may raise exception
num = int("string")
except ValueError as ve:
raise TypeError("A type error occurred") from ve
Context Managers for Exception Handling
Context managers simplify common resource management patterns by encapsulating standard uses of try statement/except/finally in so-called context management protocols.
with open('file.txt', 'r') as file:
content = file.read()
Remember:
Exception handling is crucial for building robust and reliable Python applications. Embrace these techniques to confidently confront errors and write bug-free python code!
Frequently Asked Questions (FAQs)
What is an exception in Python?
An exception in Python is an error that occurs during the execution of a program, which disrupts the normal flow of the program’s instructions.
How do I catch exceptions in Python?
In Python, exceptions can be caught using a try-except block. Code that may raise an exception is placed within the try block, and code that will execute in case of an exception is placed within the except block.
How do I raise exceptions in Python?
Exceptions can be raised using the raise statement followed by the name of the exception, and optionally followed by an error message.
Can I create custom exceptions in Python?
Yes, you can create custom exceptions in Python by creating a new class derived from the base Exception class.
What is exception chaining in Python?
Exception chaining occurs when an exception is raised in response to catching a different exception. This is useful for capturing and retaining the traceback information of the original exception.
How do I log exceptions in Python?
You can log exceptions in Python using the logging module. By configuring a logger, you can capture exception information and write it to a log file or other output streams.
What are some common built-in exceptions in Python?
Some common built-in exceptions in Python include ValueError, TypeError, IndexError, KeyError, and FileNotFoundError.
How can I handle multiple exceptions in Python?
Multiple exceptions can be handled by using multiple except blocks with a try block or by specifying multiple exceptions in a single except block using a tuple.
What is the finally block in Python?
The finally block in Python is a block of code that will be executed no matter what, whether an exception is raised or not. It is often used for cleanup code.
What is the difference between errors and exceptions in Python?
Errors are issues in a program that the programmer does not expect to occur, while exceptions are conditions that a programmer anticipates and writes code to handle.