Python
Python, being a versatile and robust programming language, provides a myriad of data structures to accommodate different data storage and manipulation needs. Among these data structures, Lists and Sets hold a significant place due to their unique characteristics and wide range of applications. This article delves deep into the nuances of converting a list to a set in Python, a common yet crucial operation that programmers often come across.
Key Takeaways
- Efficient Conversion Methods: Utilizing Python’s in-built functions like the
set()
constructor for a hassle-free conversion.
- Unique Element Storage: Sets inherently store unique elements, filtering out duplicates from lists during conversion.
- Performance Insights: Understanding the time complexity associated with different conversion methods.
- Practical Use-Cases: Where and why you might need to convert a list to a set in Python.
Python offers various methods to convert a list to a set, each with its own set of advantages and suitable use-cases. Here’s a breakdown of some of the prominent methods:
Method 1: Utilizing the set()
Constructor
The set()
constructor is the most straightforward method to convert a list to a set in Python. It’s not only easy to use but also the fastest among the methods discussed here.
Procedure
- Define or obtain the list you want to convert.
- Call the
set()
constructor, passing the list as an argument.
- The
set()
constructor returns a new set containing all the unique elements from the list.
Example:
# Define the list
my_list = [1, 2, 3, 4, 5, 5, 6, 7]
# Convert the list to a set using set() constructor
my_set = set(my_list)
# Output: {1, 2, 3, 4, 5, 6, 7}
print(my_set)
Benefits:
- Simplicity: The
set()
constructor provides a simple and readable way to convert a list to a set.
- Performance: It’s the fastest method for conversion with a time complexity of O(N), where N is the number of elements in the list
Applications:
- When you have a list with duplicate elements and you want to obtain a set of unique elements.
- In scenarios where performance is a key concern, using the
set()
constructor is advisable.
Method 2: Employing For Loop and set.add()
method
Another effective way to convert a list to a set is by employing a for loop along with the set.add()
method. This method is more verbose but provides a clear step-by-step process of conversion.
Procedure
- Initialize an empty set.
- Iterate through the list using a for loop.
- In each iteration, add the current element to the set using the
set.add()
method.
Example:
# Define the list
my_list = [1, 2, 3, 4, 5, 5, 6, 7]
# Initialize an empty set
my_set = set()
# Use a for loop to add each element to the set
for element in my_list:
my_set.add(element)
# Output: {1, 2, 3, 4, 5, 6, 7}
print(my_set)
Benefits:
- Transparency: Each step of the conversion process is explicitly laid out, making it easy to understand.
- Flexibility: Provides room for additional logic during conversion, such as condition-based filtering.
Applications:
- When you want more control over the conversion process, or need to add conditional logic during conversion.
Other Conversion Techniques
There are other nuanced methods to convert a list to a set in Python, each with its unique benefits. These include:
- Set Comprehension: A compact and Pythonic way to convert a list to a set, especially useful in scenarios where you want to apply some conditions or transformations to the list elements during conversion.
- Using
dict.fromkeys()
: An indirect method to convert the list to a set by first converting the list to a dictionary and then to a set.
Table 1: Performance Comparison of Different Conversion Methods
Method |
Time Complexity |
Execution Time |
set() Constructor |
O(N) |
0.00016019 |
For Loop and add() |
O(N) |
0.00019580 |
Set Comprehension |
O(N) |
Not Provided |
dict.fromkeys() |
O(N) |
Not Provided |
The above table elucidates the time complexity and execution time associated with each method, providing a glimpse into the performance aspects of these conversion techniques.
<2 class="labelbar">Method 3: Utilizing Set Comprehension
Set comprehension offers a compact syntax to convert a list to a set, which is especially handy when you want to apply conditions or transformations to the list elements during the conversion process.
Procedure:
- Define or obtain the list to be converted.
- Utilize set comprehension syntax to iterate through the list, applying any desired conditions or transformations.
- The result is a new set containing the processed elements from the list.
Example:
# Define the list
my_list = [1, 2, 3, 4, 5, 5, 6, 7]
# Convert the list to a set using set comprehension
my_set = {x for x in my_list}
# Output: {1, 2, 3, 4, 5, 6, 7}
print(my_set)
Benefits:
- Compactness: Set comprehension provides a more compact and readable syntax.
- Condition-based Conversion: Easily incorporate conditions or transformations during the conversion process.
Applications:
- Useful when you want to filter or transform elements while converting the list to a set.
Method 4: Employing dict.fromkeys()
This method is an indirect way to convert a list to a set by first converting the list to a dictionary using the dict.fromkeys()
method, and then converting the resulting dictionary to a set.
Procedure:
- Define or obtain the list to be converted.
- Use the
dict.fromkeys()
method to convert the list to a dictionary.
- Convert the resulting dictionary to a set.
Example:
# Define the list
my_list = [1, 2, 3, 4, 5, 5, 6, 7]
# Convert the list to a dictionary using dict.fromkeys()
temp_dict = dict.fromkeys(my_list)
# Convert the dictionary to a set
my_set = set(temp_dict)
# Output: {1, 2, 3, 4, 5, 6, 7}
print(my_set)
Benefits:
- Unique Element Assurance: Ensures that only unique elements are transferred to the set, as dictionaries do not allow duplicate keys.
- Indirect Conversion: Provides a roundabout way to achieve list to set conversion, which might be useful in specific scenarios.
Applications:
- When the direct methods of conversion are not suitable or when an intermediary dictionary is desirable for some reason.
Frequently Asked Questions (FAQs)
Here are some commonly asked questions regarding the conversion of lists to sets in Python:
- What is the time complexity of converting a list to a set?
- The time complexity is O(N), where N is the number of elements in the list.
- How to handle a list of lists while converting to a set?
- What happens to duplicate elements during conversion?
- Duplicate elements are automatically removed during conversion, as sets only store unique elements.
- How to convert a list to a set based on a condition?
- Utilize set comprehension or a for loop with a condition to filter elements while converting the list to a set.
- Are there any performance differences among various conversion methods?
- Yes, the
set()
constructor method is generally faster compared to other methods, but the difference might not be significant for small lists.
Python
Writing lists to files in Python is a fundamental skill that every programmer should master. It not only forms the basis for file operations but also opens the door to data serialization and storage. In this comprehensive guide, we delve into various methods of writing lists to files in Python, each with its unique advantages, use-cases, and syntax.
- Key Takeaways:
- Understanding the syntax and use-cases of different methods for writing lists to files.
- Grasping the role of loops in iterating through list items during file writing.
- Discerning the benefits of using JSON and Pickle modules for list serialization.
- Insights into the
io
module for streamlined file operations.
Utilizing Basic Write Methods
Python offers a couple of straightforward methods to write lists to files. The most common among these are the write()
and writelines()
methods. These methods are part of the built-in file
object in Python.
The write()
Method
The write()
method is a basic method that allows for writing strings to files. However, when it comes to writing lists, a little more effort is required. Each item in the list needs to be converted to a string, and then written to the file individually.
# Example usage of write() method
my_list = ["apple", "banana", "cherry"]
with open("output.txt", "w") as file:
for item in my_list:
file.write(item + "\n")
In this example, we open a file named output.txt
in write mode (w
). We then iterate through my_list
, writing each item to the file followed by a newline character to ensure each item appears on a new line in the file.
- Advantages:
- Simple and straightforward.
- Provides granular control over the file writing process.
- Disadvantages:
- Can be verbose for large lists.
- Requires manual iteration through the list.
The writelines()
Method
The writelines()
method is somewhat more suited for writing lists to files as it can write a list of strings to a file in a single call. However, it does not add newline characters, so you might still need to iterate through the list to format it correctly.
# Example usage of writelines() method
my_list = ["apple", "banana", "cherry"]
formatted_list = [item + "\n" for item in my_list]
with open("output.txt", "w") as file:
file.writelines(formatted_list)
In this example, we first format my_list
to ensure each item ends with a newline character. We then write the formatted list to output.txt
using writelines()
.
- Advantages:
- Disadvantages:
- Does not add newline characters automatically.
- May require additional formatting of the list before writing.
Engaging Loops for Iterative Writing
Loops, especially the for
loop, play a crucial role in writing lists to files in Python. They provide the mechanism to iterate through each item in the list and write it to the file.
# Example usage of for loop for writing lists to files
my_list = ["apple", "banana", "cherry"]
with open("output.txt", "w") as file:
for item in my_list:
file.write(item + "\n")
In this code snippet, we utilize a for
loop to iterate through my_list
, writing each item to output.txt
. This method provides granular control over the file writing process, ensuring each item is written as desired.
- Advantages:
- Provides granular control over the file writing process.
- Allows for custom formatting of list items before writing.
- Disadvantages:
- Can be verbose for large lists.
Exploring the JSON and Pickle Modules for Serialization
When it comes to writing lists to files in Python, serialization is a concept that often comes into play. Serialization refers to the process of converting data structures or object states into a format that can be stored or transmitted and reconstructed later. In Python, the json
and pickle
modules provide serialization and deserialization functionalities for various data structures, including lists.
JSON Serialization
The json
module in Python provides methods for working with JSON data, including serializing Python liststo JSON format.
# Example usage of json module for writing lists to files
import json
my_list = ["apple", "banana", "cherry"]
with open("output.json", "w") as file:
json.dump(my_list, file)
This example demonstrates how to write a Python list to a file in JSON format using the json.dump()
method.
- Advantages:
- Human-readable format.
- Wide support across various languages and platforms.
- Disadvantages:
- Not suitable for complex Python objects.
Pickle Serialization
Unlike the json
module, the pickle
module can handle a wider range of Python data types during serialization.
# Example usage of pickle module for writing lists to files
import pickle
my_list = ["apple", "banana", "cherry"]
with open("output.pkl", "wb") as file:
pickle.dump(my_list, file)
Here, we use the pickle.dump()
method to write my_list
to a file in pickle format.
- Advantages:
- Can handle complex Python data types.
- Suitable for Python-specific applications.
- Disadvantages:
- Not human-readable.
- Limited support outside Python environments.
Leveraging the io
Module for Streamlined File Operations
The io
module in Python provides a plethora of tools for working with streams – sequences of data elements made available over time. These tools are indispensable when it comes to reading and writing lists to files in Python.
Basics of the io
Module
The io
module provides the basic interfaces to stream I/O. Among these, the io.StringIO
and io.BytesIO
classes are the most noteworthy for file operations with text and binary files respectively.
# Example usage of io.StringIO for writing lists to files
import io
my_list = ["apple", "banana", "cherry"]
buffer = io.StringIO()
for item in my_list:
buffer.write(item + "\n")
# Get the content from the buffer and write to a file
with open("output.txt", "w") as file:
file.write(buffer.getvalue())
In this example, an io.StringIO
buffer is used to write the list items, which are then written to a file.
- Advantages:
- Efficient memory usage for large lists.
- Streamlined handling of string data.
- Disadvantages:
- Might be overkill for simple file operations.
- Requires an understanding of buffer management.
Practical Implementations and Performance Considerations
When it comes to practical implementations, choosing the right method to write lists to files in Python depends on the specific requirements of your project. Performance considerations, especially for large lists, are crucial.
- Performance Considerations:
- For large lists, using buffer-based or streaming methods can be more efficient.
- Serializing complex data structures with Pickle or similar modules can have a performance impact.
- Error Handling:
Frequently Asked Questions
- What are the differences between the
write()
and writelines()
methods?
- The
write()
method writes a string to a file, while the writelines()
method writes a list of strings. However, writelines()
does not add newline characters automatically.
- Can I use a for loop to write a list to a file in Python?
- Yes, a for loop can be used to iterate through the items in a list and write each item to a file.
- What are the benefits of using the JSON and Pickle modules for writing lists to files?
- JSON provides a human-readable format with wide support across various languages, while Pickle can handle complex Python data types, making it suitable for Python-specific applications.
- How can I use the
io
module to write a list to a file in Python?
- The
io
module provides StringIO
and BytesIO
classes that can be used to create stream objects. These objects can be used to write list items to a buffer, which can then be written to a file.
- Are there other modules or methods for writing lists to files in Python?
- Yes, besides the mentioned methods, other modules like
csv
for CSV file writing or custom serialization libraries can also be used.
Python
In the vast and versatile world of programming, Python stands out with its ease of learning and a wide range of applications. One fundamental concept in Python, and programming in general, is handling lists. Specifically, prepending a list or adding elements to the beginning of a list is a common operation. However, unlike appending, which is straightforward in Python, prepending requires a bit more attention.
Key Takeaways:
- Understanding various methods to prepend a list in Python.
- Grasping the performance and memory implications of each method.
- Identifying the scenarios where one method is preferable over the others.
Understanding Python List Prepending
Python provides multiple ways to prepend a list, each with its unique advantages and disadvantages. This flexibility allows developers to choose the method that best suits their specific scenario. The methods we will explore include:
- Using the
insert()
method
- Utilizing the
+
operator
- Applying list slicing
- Employing
collections.deque.appendleft()
method
- Reverse-Append-Reverse method
- Sorting method
In the subsequent sections, we delve deeper into each of these methods, shedding light on their syntax, performance, and suitable use cases.
Diving into Prepending Methods
Using the insert()
Method
The insert()
method is a straightforward way to prepend a list in Python. It requires specifying the index where the new element should be inserted, and for prepending, this index is 0. Here’s a simple demonstration:
my_list = [2, 3, 4]
my_list.insert(0, 1)
print(my_list) # Output: [1, 2, 3, 4]
Advantages:
- Easy to use and understand.
- Suitable for small lists due to its simplicity.
Disadvantages:
- Not efficient for large lists as it has to shift all other elements by one position, making it a linear time operation, O(n).
Utilizing the +
Operator
The +
operator is another intuitive method to prepend a list. It involves concatenating the new element, wrapped in a list, with the original list. Here’s how you can do it:
my_list = [2, 3, 4]
my_list = [1] + my_list
print(my_list) # Output: [1, 2, 3, 4]
Advantages:
- Readable and self-explanatory code.
Disadvantages:
- Similar to the
insert()
method, the +
operator is not efficient for large lists as it creates a new list, requiring additional memory.
Applying List Slicing
List slicing in Python is a powerful feature that can also be used to prepend a list. By specifying a slice that encompasses the entire list, you can assign a new value to the beginning of the list. Here’s a demonstration:
my_list = [2, 3, 4]
my_list[:0] = [1]
print(my_list) # Output: [1, 2, 3, 4]
Advantages:
Disadvantages:
- The syntax might be confusing for beginners.
These methods form the basis of list prepending in Python, each catering to different scenarios and performance considerations. Whether you are dealing with small lists or large datasets, understanding the nuances of these methods will enable you to write efficient and readable code.
Performance and Memory Implications
When it comes to choosing a method for prepending a list, performance, and memory efficiency are crucial factors to consider. Let’s delve into a comparative analysis of the methods discussed:
Method |
Time Complexity |
Space Complexity |
Suitable for Large Lists |
insert() method |
O(n) |
O(1) |
No |
+ operator |
O(n) |
O(n) |
No |
List slicing |
O(n) |
O(n) |
No |
collections.deque |
O(1) |
O(1) |
Yes |
Reverse-Append-Reverse |
O(n) |
O(n) |
No |
Sorting method |
O(n log n) |
O(n) |
No |
The collections.deque.appendleft()
method shines with its constant time and space complexity, making it a viable option for large lists.
Advanced Prepending Techniques
Prepending in Circular Linked Lists
In specific scenarios, especially when dealing with large datasets, traditional list prepending methods may not be the most efficient. This is where data structures like Circular Linked Lists come into play. Circular Linked Lists provide a more complex, yet efficient way to prepend elements, especially in scenarios where the data is being continuously added and removed.
Using a Python List Subclass with O(1) Prepend
Creating a subclass of the Python list with a method to handle O(1) prepend operations can also be a viable solution. This advanced technique allows for efficient prepending, especially in performance-critical applications.
Exploring Alternative Data Structures for Prepending
When working with larger datasets or in performance-critical applications, the conventional methods of list prepending in Python may not suffice. In such cases, alternative data structures may prove to be more efficient and suitable. Here, we delve into some of these alternatives and compare them with the standard list prepending methods.
Employing collections.deque
for Efficient Prepending
The collections.deque
(double-ended queue) is a built-in Python data structure that allows for efficient appending and prepending of elements with O(1) time complexity. Here’s a simple demonstration of how to use deque
to prepend a list:
from collections import deque
my_list = deque([2, 3, 4])
my_list.appendleft(1)
print(list(my_list)) # Output: [1, 2, 3, 4]
Advantages:
- Highly efficient for both small and large lists.
- Constant time complexity for prepending, O(1).
Disadvantages:
Leveraging Linked Lists for Prepending
Linked lists are another alternative that provides efficient prepending. In a linked list, each element points to the next element, making it easy to insert elements at the beginning of the list.
class Node:
def __init__(self, value=None):
self.value = value
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def prepend(self, value):
new_node = Node(value)
new_node.next = self.head
self.head = new_node
# Usage:
my_list = LinkedList()
my_list.prepend(1)
Advantages:
- Constant time complexity for prepending, O(1).
- Suitable for scenarios with frequent insertions and deletions.
Disadvantages:
- More complex than using built-in list methods.
- Not native to Python; requires implementation.
Frequently Asked Questions
- How can I prepend multiple elements to a list in Python?
- Multiple elements can be prepended using list slicing or the
extendleft()
method of collections.deque
.
- What are the performance implications of different prepend methods?
- Methods like
insert()
and the +
operator have linear time complexity, making them less suitable for large lists. On the other hand, collections.deque
provides constant time complexity for prepending.
- How does prepending affect the order of the list?
- Prepending adds elements to the beginning of the list, shifting the existing elements to the right.
- Is there a built-in
prepend()
method in Python?
- No, Python does not have a built-in
prepend()
method. However, you can use other methods like insert()
or collections.deque.appendleft()
to achieve the same result.
- When should I use alternative data structures for prepending?
- Alternative data structures like
collections.deque
or linked lists are suitable when dealing with large datasets or in performance-critical scenarios where the standard list prepending methods are inefficient.
Python
In the realm of data manipulation and analysis, list subtraction is a crucial operation that allows developers to derive new datasets from existing ones. Python, known for its robust data handling capabilities, provides multiple avenues for performing list subtraction efficiently and effectively.
Key Takeaways:
- Understanding and implementing list subtraction in Python.
- Different methods of list subtraction including list comprehension, set operations, using loops, and library functions.
- Performance comparison of various list subtraction methods.
- Common mistakes and pitfalls to avoid during list subtraction.
- Advanced techniques for element-wise subtraction and library support for list subtraction.
Methods for List Subtraction
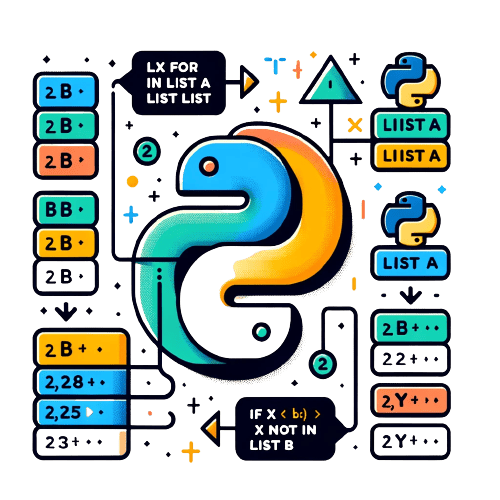
List Comprehension
List comprehension is a compact way to process all the elements in a list. It’s often appreciated for its readability and concise syntax. Below is an example of list subtraction using list comprehension:
a = [1, 2, 3, 4, 5]
b = [4, 5]
result = [item for item in a if item not in b]
# result: [1, 2, 3]
In the above code, we created a new list result
, which contains elements that are in list a
but not in list b
List Comprehension.
Set Operations
Set operations provide another method for list subtraction. The set()
function can be used to convert lists to sets, perform set subtraction, and then convert the result back to a list, as shown below:
a = [1, 2, 3, 4, 5]
b = [4, 5]
result = list(set(a) - set(b))
# result: [1, 2, 3]
In this example, we first converted lists a
and b
to sets, performed the subtraction operation using the -
operator, and then converted the result back to a listSubtract Two Lists.
Using Loops
Loops offer a more traditional way of performing list subtraction. Here’s how you can do it using a for loop:
a = [1, 2, 3, 4, 5]
b = [4, 5]
result = []
for item in a:
if item not in b:
result.append(item)
# result: [1, 2, 3]
In the code above, we iterated through list a
and appended each element not present in list b
to the result list.
Library Functions
Python has several library functions that can aid in list subtraction. The numpy library, for instance, provides straightforward ways to perform element-wise subtraction between two lists:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
b = np.array([1, 1, 1, 1, 1])
result = a - b
# result: array([0, 1, 2, 3, 4])
In this code snippet, we used the numpy
library to perform element-wise subtraction of two listsPerform List Subtraction in Python.
Performance Comparison
It’s crucial to understand the performance implications of different list subtraction methods, especially when working with large datasets. Here’s a summary of the performance of each method discussed:
Method |
Time Complexity |
Space Complexity |
List Comprehension |
O(n) |
O(n) |
Set Operations |
O(n) |
O(n) |
Using Loops |
O(n^2) |
O(n) |
Library Functions |
O(n) |
O(n) |
The table shows that while all methods have a linear space complexity of O(n), using loops has a time complexity of O(n^2) due to the nested operation of checking the existence of an element in another list.
Common Mistakes and Pitfalls
When performing list subtraction in Python, it’s easy to overlook certain aspects that could potentially lead to incorrect results or performance bottlenecks. Here are some common pitfalls to avoid:
Advanced List Subtraction Techniques
Element-wise Subtraction
Element-wise subtraction refers to the subtraction of corresponding elements from two lists. This method requires the two lists to be of the same length. Here’s how you can perform element-wise subtraction using the zip
function and a list comprehension:
a = [1, 2, 3, 4, 5]
b = [1, 1, 1, 1, 1]
result = [item_a - item_b for item_a, item_b in zip(a, b)]
# result: [0, 1, 2, 3, 4]
In this example, the zip
function is used to pair up the corresponding elements from lists a
and b
, and the list comprehension is used to subtract the paired elements.
Library Support
Various libraries in Python provide support for list subtraction. One such library is NumPy, which offers a simple and effective way to perform list subtraction:
import numpy as np
a = np.array([1, 2, 3, 4, 5])
b = np.array([1, 1, 1, 1, 1])
result = a - b
# result: array([0, 1, 2, 3, 4])
In the code snippet above, we utilized the NumPy library to perform element-wise subtraction between two lists, resulting in a new list with the subtracted valuesPerform List Subtraction in Python.
Real-world Applications of List Subtraction
List subtraction is a versatile operation used in numerous real-world applications. Some of these include:
- Data Cleaning: Removing specific values or outliers from a dataset to ensure accurate analysis.
- Feature Engineering: Deriving new features from existing data for machine learning models.
- Inventory Management: Subtracting sold items from the inventory list to keep it updated.
These applications highlight the importance and utility of list subtraction in Python for solving real-world problems.
Frequently Asked Questions
- Why is list comprehension a preferred method for list subtraction in Python?
- List comprehension is preferred due to its readability, concise syntax, and relatively better performance compared to using loops for list subtraction.
- How do set operations differ from list comprehension in list subtraction?
- Set operations involve converting lists to sets, performing set subtraction, and then converting the result back to a list. This method is efficient but will remove duplicate elements, unlike list comprehension.
- Are there any libraries in Python specifically designed for list subtraction?
- While there isn’t a library specifically for list subtraction, libraries like NumPy provide functions that can be used for list subtraction.
- How does element-wise subtraction differ from other list subtraction methods?
- Element-wise subtraction involves subtracting corresponding elements from two lists, whereas other methods involve subtracting the entirety of one list from another.
- Can list subtraction be performed on lists with different data types?
- It’s advisable to have consistent data types in the lists to avoid unexpected behaviours during the subtraction operation.
Python
FFmpeg is a remarkable piece of software used for handling multimedia data. It provides command-line tools and libraries for handling, converting, and streaming audio and video. On the other hand, Python, a high-level, general-purpose programming language, is known for its ease of use and versatility. When combined, FFmpeg and Python empower developers to work with multimedia data seamlessly, and one library that facilitates this is ffmpeg-python.
Key Takeaways:
- Understanding the installation and basic configuration of FFmpeg.
- Learning basic and advanced operations.
- Gaining insights into optimizing performance while handling large multimedia files.
- Exploring real-world applications of using this library.
Setting Up ffmpeg python
Installation Process
Getting up and running is straightforward. It can be installed using pip:
pip install ffmpeg-python
For more detailed instructions, you can visit the official FFmpeg on PyPi.
Basic Configuration
Once installed FFmpeg, configuring to suit your project needs is the next step. This involves setting up paths, understanding basic commands, and getting familiar with the library’s syntax.
Basic Operations
FFmpeg provides an elegant and powerful API for accessing FFmpeg’s capabilities. Here are some basic operations you can perform:
Reading and Writing Video Files
Extracting Audio, Video, and Other Streams
- Separating audio and video streams from a multimedia file.
- Extracting metadata from multimedia files.
Key Methods & Functions Guide
1. .input()
This method is used to specify the input source, which can be a file, stream, or other sources.
Usage:
stream = ffmpeg.input('path/to/input/file.mp4')
2. .output()
Designate the output destination for the processed data. This method also allows specifying codecs, formats, and other encoding parameters.
Usage:
ffmpeg.output(stream, 'path/to/output/file.mp4')
3. .run()
Execute the FFmpeg command with the specified input, filters, and output parameters. This method runs the constructed FFmpeg command in the background.
Usage:
ffmpeg.run(stream)
4. .filter()
This method applies a filter to the input stream. Filters can be used for tasks like resizing, cropping, and other transformations.
Usage:
filtered_stream = stream.filter('crop', out_w=640, out_h=360)
5. .overwrite_output()
By using this method, you can set FFmpeg to overwrite any existing files with the same name as the specified output.
Usage:
ffmpeg.output(stream, 'path/to/output/file.mp4').overwrite_output()
6. .view()
This method is particularly useful for debugging. It helps visualize the constructed filter graph.
Usage:
stream.view(filename='filter_graph.png')
Advanced Operations
Complex Filtering Examples
FFmpeg excels when it comes to complex filtering of audio and video data. It provides a plethora of built-in filters and also allows for custom filter graphs.
Working with Streaming Data
- Reading from and writing to streaming sources.
- Handling live streaming data with FFmpeg.
Performance Optimization Tips
Handling large multimedia files and streams efficiently is crucial for performance. Here are some tips:
Improving Processing Speed
- Utilizing multi-threading.
- Optimizing filter graphs.
Handling Large Multimedia Files
Real-World Applications
FFmpeg finds its applications in various real-world scenarios:
Use Cases in Different Industries
- Video editing platforms.
- Real-time video processing in surveillance.
- Automated media processing pipelines.
Notable Projects Utilizing ffmpeg Library
Various projects and platforms leverage FFmpeg for multimedia processing. For instance, the FFmpeg GitHub repository showcases several projects utilizing this library.
Comparing FFmpeg with Other Libraries
Pros and Cons
- Pros: Easy integration with FFmpeg, robust filtering capabilities, active community support.
- Cons: Might require a steep learning curve for beginners, dependent on FFmpeg.
Performance Comparison
Comparing FFmpeg
with other libraries like moviepy
or pydub
in terms of performance, ease of use, and community support.
Community and Support
The FFmpeg
community plays a pivotal role in its growth and development. Being open-source, it thrives on collective contributions and support.
GitHub Repository and Community Engagement
The FFmpeg GitHub repository is a hub for developers to discuss issues, propose enhancements, and contribute to the codebase. The active involvement of the community ensures that FFmpeg
remains up-to-date with the latest multimedia processing trends.
Available Resources for Learning and Troubleshooting
There’s a wealth of resources available for learning FFmpeg
. Apart from the official documentation, there are tutorials, blog posts, and forums dedicated to helping developers troubleshoot issues and understand the library better.
Tables with Relevant Facts
Feature |
Description |
Installation |
Easy installation with pip |
Documentation |
Extensive documentation available |
Community Support |
Active community on GitHub |
Performance |
High performance with optimized memory management |
Filtering Capabilities |
Robust filtering with built-in and custom filters |
Frequently Asked Questions
What is FFmpeg in Python?
FFmpeg in Python is a Pythonic interface for executing FFmpeg commands, allowing manipulation of multimedia files like video and audio conversion, cutting and trimming, and more.
How do I install the FFmpeg Python package?
You can install the FFmpeg Python package using pip: run ‘pip install ffmpeg-python’ in your command prompt.
Can I use FFmpeg to trim videos in Python?
Yes, you can trim videos using FFmpeg in Python by specifying the start and duration of the trim in the FFmpeg commands.
Is it possible to get the width and height of a video using FFmpeg in Python?
Yes, you can get the width and height of a video by probing the video’s metadata using FFmpeg in Python.
How can I save a thumbnail from a video using FFmpeg in Python?
You can generate a thumbnail from a video with FFmpeg in Python by specifying the time and scaling options in your command.
Can I flip a video horizontally or vertically using FFmpeg in Python?
Yes, you can flip videos horizontally or vertically in Python using the ‘ffmpeg.hflip()’ and ‘ffmpeg.vflip()’ commands, respectively.
What kind of operations can be performed with FFmpeg in Python?
FFmpeg in Python can be used for a wide range of operations including video/audio format conversion, compression, extraction, and more.
How do I use FFmpeg to resize a video and apply a watermark in Python?
You can resize a video and apply a watermark in Python using FFmpeg by specifying the scale filter and overlay filter in the command.
Is FFmpeg in Python suitable for multimedia file manipulation?
Yes, FFmpeg is a powerful tool in Python for various multimedia file operations, making it suitable for comprehensive multimedia applications.
Are there any alternatives to FFmpeg for video editing in Python?
Bannerbear is an alternative to FFmpeg that offers easy integration into platforms or apps to generate designed visual content, including videos, automatically.
Python
In the realm of programming, making decisions based on certain conditions is a core aspect of writing effective and functional code. Python, a highly intuitive and powerful programming language, offers a range of operators and statements to aid in this process. Among these, the combination of if statement and not operator, expressed as if not, is a potent duo that provides developers with a robust mechanism for handling conditional logic. This article dives into the intriguing world of if not in Python, shedding light on its syntax, usage, and real-world applications.
Key Takeaways:
- Understanding the synergy between if and not in Python.
- Grasping the syntax and common usage scenarios of if not.
- Exploring Boolean and Non-Boolean contexts.
- Uncovering best practices and real-world applications.
- Delving into Frequently Asked Questions surrounding if not.
Introduction to Python if not
Python’s if statement is a fundamental building block that allows for conditional execution of code. It evaluates a condition, and if the condition is true, the code within the block is executed. On the flip side, the not operator is a logical operator that reverses the truth value of the condition it precedes. When used together, if not provides a means to execute code if a particular condition is false.
Syntax and Basic Usage
The syntax of if not is straightforward. It combines the if statement with the not operator to form a condition that is negated. Here’s a simple illustration of its syntax:
if not condition:
statement(s)
In the following example, the if not statement is used to check whether a number is less than zero, and if so, a message is printed to the console:
while True:
user_input = input("Enter 'q' to quit: ")
if not user_input != 'q':
break
Output:
The number is negative
This simple example showcases the essence of if not in action – negating a condition and executing code based on that negation.
Exploring Boolean Contexts
The utility of if not extends beyond simple condition negation. It finds a significant application in Boolean contexts, where it can be employed to check for truthiness or falsiness of values, and act accordingly.
Usage with while Loops
In Python, the while loop continues execution as long as a specified condition remains true. However, with the use of if not, you can create loops that continue execution until a certain condition becomes false. This is particularly useful in scenarios where you need to monitor a particular state or wait for a certain condition to cease.
while True:
user_input = input("Enter 'q' to quit: ")
if not user_input != 'q':
break
In this snippet, the if not statement is used to break out of the while loop once the user enters ‘q’.
Testing for Membership
Python’s if not can also be employed to test for membership within collections such as lists, tuples, or dictionaries.
my_list = [1, 2, 3, 4, 5]
if not 6 in my_list:
print("6 is not in the list")
Output:
6 is not in the list
This usage of if not allows for intuitive and readable code when testing for the absence of a particular value within a collection.
Non-Boolean Contexts and Best Practices
The journey with if not does not end with Boolean contexts; it finds relevance in Non-Boolean contexts too, presenting a versatile tool in a developer’s arsenal.
Function-Based not Operator
Python also facilitates a function-based approach to the not operator, which can be handy in certain scenarios, especially when dealing with complex conditions or when needing to negate the output of function calls.
def is_positive(num):
return num > 0
if not is_positive(-5):
print("The number is not positive")
Output:
The number is not positive
This snippet demonstrates a function-based approach to utilizing if not, showcasing its adaptability.
Best Practices
Adhering to best practices when using if not can lead to cleaner, more readable code. Some recommended practices include:
- Avoiding double negatives as they can lead to code that is hard to understand.
- Being explicit in your conditions to ensure code readability and maintainability.
- Using parentheses to clarify order of operations in complex conditions.
Real-world Applications
The practical applications of if not are vast and varied, spanning across different domains and use cases.
- Simplifying Conditional Statements: if not can significantly simplify conditional logic, making code more readable and maintainable.
- Input Validation: It is instrumental in validating user input, ensuring that undesirable or incorrect values are properly handled.
Delving into the Syntax and Usage
Understanding the syntax and common usage scenarios of if not is crucial for effectively leveraging this operator in your Python projects.
Syntax of if not
The if not syntax is a fusion of the if statement with the not operator, forming a negated conditional statement. Here’s a refresher on its syntax:
if not condition:
statement(s)
Common Use Cases
age = 15
if not age >= 18:
print("You are not eligible to vote")
- Negating Conditions: Negating a condition to execute code when a condition is false.
- Checking for Empty Collections: Verifying if a collection like a list, tuple, or dictionary is empty.
my_list = []
if not my_list:
print("The list is empty")
- Validating User Input: Ensuring that the user input meets certain criteria.
user_input = input("Enter a positive number: ")
if not user_input.isdigit():
print("Invalid input. Please enter a positive number")
Advanced Usage and Best Practices
Taking if not to the next level involves exploring its utility in non-Boolean contexts and adhering to best practices for clean, efficient code.
Non-Boolean Contexts
In non-Boolean contexts, if not can test the truthiness of values that aren’t strictly True or False. For instance, it treats empty strings, empty collections, and zero values as False.
user_input = input("Enter a positive number: ")
if not user_input.isdigit():
print("Invalid input. Please enter a positive number")
Best Practices
- Avoiding Double Negatives: Steer clear of double negatives as they can muddle the code’s readability.
# Avoid this
if not age != 18:
print("Age is 18")
# Prefer this
if age == 18:
print("Age is 18")
- Explicit Conditions: Strive for explicit conditions to promote code clarity and maintainability.
# Avoid this
if not is_logged_in:
print("Please log in")
# Prefer this
if is_logged_in == False:
print("Please log in")
Practical Applications
The real-world applications of if not are boundless, offering a versatile tool for various programming scenarios.
- Error Handling:if not can be instrumental in error handling by checking for undesirable states.
# Avoid this
if not is_logged_in:
print("Please log in")
# Prefer this
if is_logged_in == False:
print("Please log in")
- Data Validation: It is invaluable for validating data, ensuring that the data meets certain criteria before proceeding.
# Avoid this
data = get_data()
if not data:
print("No data received. Cannot proceed.")
- Control Flow:
if not
is a key player in managing the control flow of a program, helping to direct the execution of code based on specified conditions.
These practical applications underscore the value and versatility of the if notoperator in Python, illuminating its capability to simplify and streamline conditional logic in your code. Through a thorough understanding and prudent usage of if not, you can write cleaner, more efficient, and more intuitive code in your Python projects.
Frequently Asked Questions
What does the 'if not' statement do in Python?
In Python, the ‘if not’ statement is used to check if a condition is False. If the condition is False, the code block under ‘if not’ will be executed.
Can 'if not' be used with Boolean values?
Yes, ‘if not’ can be used with Boolean values. It reverses the Boolean value, executing the code block if the value is False.
How does 'if not' work with lists in Python?
‘If not’ checks if a list is empty in Python. If the list is empty, the statement after ‘if not’ gets executed.
Is 'if not' different from 'if' in Python?
‘If not’ in Python is used for negation, checking if a condition is False, whereas ‘if’ checks if a condition is True.
Can 'if not' be combined with 'else' in Python?
Yes, ‘if not’ can be used with an ‘else’ clause in Python. The ‘else’ block executes when the ‘if not’ condition evaluates to False.
How can 'if not' be used with variables?
‘If not’ can be used to check if a variable is None, empty, or False in Python. If the variable meets any of these conditions, the ‘if not’ block will execute.
Can 'if not' be used with strings?
Yes, ‘if not’ can check if a string is empty in Python. It executes the code block if the string is empty.
What is the purpose of using 'if not' in Python?
‘If not’ is used in Python to improve readability and succinctly express a condition that checks for falseness.
Can 'if not' be nested in Python?
Yes, ‘if not’ statements can be nested within other ‘if’ or ‘if not’ statements in Python for more complex conditional logic.
Is 'if not' a common Pythonic way to express conditions?
Yes, using ‘if not’ is a Pythonic way to express conditions that check for False, None, or empty values.