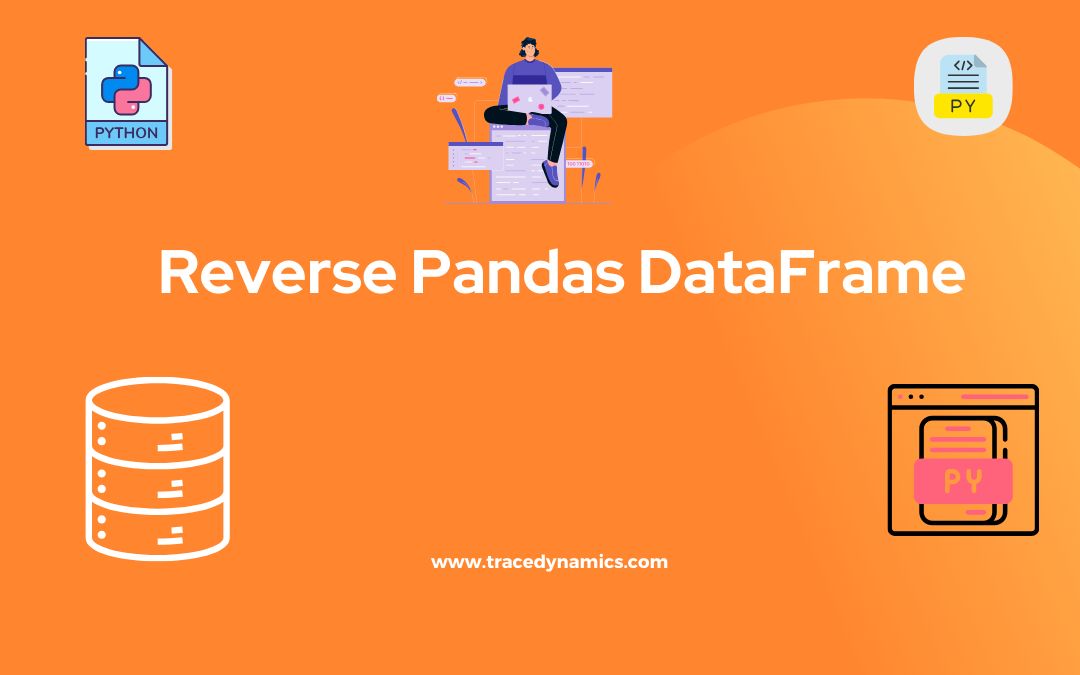
Reverse Pandas: DataFrame Reversal in Python
Reverse Pandas: Pandas is a powerful library in Python, widely used for data manipulation and analysis. It provides high-performance, easy-to-use data structures, among which DataFrame is a primary one. A common task that data analysts or data scientists might face is reversing a DataFrame, which can be crucial for certain data processing tasks.
Key Takeaways:
- Reversing a DataFrame in Pandas can be done through multiple methods like slicing, using the
reindex
method, and more. - Reversed DataFrames can be beneficial in various real-world scenarios for better data analysis and visualization.
- Several YouTube tutorials provide step-by-step guidance on how to reverse a DataFrame in Pandas.
Why Reverse a DataFrame?
Reversing a DataFrame in Pandas means altering the order of rows, columns, or both. This can be beneficial in various scenarios:
- Preparing the data for certain types of analysis or visualization.
- Making the data more readable or understandable.
- Aligning the data structure to meet certain algorithm requirements.
It’s essential to understand the need for reversing a DataFrame to better grasp the methods and techniques involved in accomplishing this task.
Methods to Reverse a DataFrame
There are several methods to reverse a DataFrame in Pandas. Each method has its use-case, and the choice of method might depend on the specific requirements of your task.
Slicing
Slicing is a straightforward method to reverse the order of rows in a DataFrame. By using the slicing syntax df[::-1]
or df.loc[::-1]
, you can easily reverse the order of rows.
- Simple and readable: This method is simple and clear in its intent.
- No additional arguments required: Slicing doesn’t require any additional arguments, making it a concise method.
Using the reindex
Method
The reindex
method is another way to reverse a DataFrame. It allows you to specify a new index for your DataFrame, and by providing a reversed index, you can reverse the order of rows.
- Flexibility:
reindex
provides more flexibility as you can specify a custom index. - Handling missing values: If there are any missing values in the new index,
reindex
will fill them with NaN values, ensuring the integrity of your data.
Reverse Panda DataFrame Examples
Basic Row Reversal using Slicing:
import pandas as pd
# Creating DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# Reversing rows
df_reversed = df[::-1]
Basic Column Reversal:
# Reversing columns
df_column_reversed = df[df.columns[::-1]]
Row Reversal using iloc:
# Reversing rows using iloc
df_iloc_reversed = df.iloc[::-1]
Column Reversal using iloc:
# Reversing columns using iloc
df_column_iloc_reversed = df.iloc[:, ::-1]
Row Reversal and Resetting Index:
# Reversing rows and resetting index
df_reset_index = df[::-1].reset_index(drop=True)
Column Reversal using loc:
# Reversing columns using loc
df_column_loc_reversed = df.loc[:, ::-1]
Row Reversal using reindex:
# Reversing rows using reindex
df_reindex_reversed = df.reindex(index=df.index[::-1])
Column Reversal using reindex:
# Reversing columns using reindex
df_column_reindex_reversed = df.reindex(columns=df.columns[::-1])
Reversing MultiIndex Levels using swaplevel:
# Creating MultiIndex DataFrame
arrays = [list('ABCD'), list('EFGH')]
index = pd.MultiIndex.from_arrays(arrays, names=('letters', 'numbers'))
multi_df = pd.DataFrame({'data': range(4)}, index=index)
# Reversing MultiIndex levels
reversed_levels_df = multi_df.swaplevel('letters', 'numbers')
Reversing DataFrame using sort_index:
# Reversing DataFrame using sort_index
df_sort_index_reversed = df.sort_index(ascending=False)
Common Mistakes and How to Avoid Them
When reversing a DataFrame in Pandas, some common mistakes could lead to incorrect results or performance issues.
- Ignoring the index: When you reverse a DataFrame, the index will also be reversed. If you want to maintain the original index, you need to reset it.
- Inefficient methods: Some methods for reversing a DataFrame might be inefficient for large DataFrames, leading to performance issues.
Advanced Reversal Techniques
Delving deeper into the world of Pandas and DataFrame manipulation, there are advanced techniques that provide more control and flexibility when it comes to reversing a DataFrame. These techniques come in handy in complex data manipulation tasks where standard reversal methods may not suffice.
Multi-Index Level Reversal
Pandas provides functionality for multi-level indexing, which is essential for higher-dimensional data analysis. Reversing the levels of a multi-index DataFrame could be achieved using the swaplevel
method.
- Syntax:
reversed_levels_df = multi_df.swaplevel('level_1', 'level_2')
- Use-case: This method is useful when you have multi-dimensional data, and you need to swap the levels for better data organization or analysis.
Reversing Column Order
In some scenarios, you might need to reverse the order of columns in a DataFrame. This could be achieved through:
- Syntax:
df[df.columns[::-1]]
- Use-case: Useful in scenarios where column order matters for data analysis or visualization.
These advanced reversal techniques offer more control over how the data is structured, aiding in complex data manipulation tasks.
Applications of Reversed DataFrames in Data Analysis
Reversed DataFrames can play a crucial role in data analysis and visualization. Below are some applications where they prove to be beneficial:
- Time Series Analysis: Reversing the order of time-stamped data for chronological analysis.
- Comparative Analysis: Comparing datasets by aligning them in a specific order.
- Data Visualization: Enhancing the readability and interpretability of visualizations by ordering the data in a particular manner.
Frequently Asked Questions
This section addresses some common queries regarding reversing a DataFrame in Pandas:
1. What is the simplest method to reverse a DataFrame in Pandas?
The simplest method to reverse a DataFrame in Pandas is by using slicing with the syntax df[::-1]
for reversing rows, or df[df.columns[::-1]]
for reversing columns.
2. How can I maintain the original index after reversing a DataFrame?
To maintain the original index after reversing a DataFrame, you can use the reset_index()
method with the syntax df_reversed = df[::-1].reset_index(drop=True)
.
3. Can I reverse a multi-index DataFrame in Pandas?
Yes, you can reverse a multi-index DataFrame in Pandas using the swaplevel()
method with the syntax reversed_levels_df = multi_df.swaplevel('level_1', 'level_2')
.
4. Are there any performance considerations when reversing a large DataFrame?
Reversing a large DataFrame may have performance implications depending on the method used. It’s advisable to test different methods on a subset of your data to gauge performance.
5. Where can I find more resources on reversing a DataFrame in Pandas?
There are several online resources available for learning how to reverse a DataFrame in Pandas.