Python
Python, a language renowned for its simplicity and elegance, offers numerous built-in modules that make coding more efficient and less error-prone. Among these is the os.path
module, specifically the os.path.join
method, an indispensable tool for any Python programmer working with file and directory paths. This article delves deep into the nuances of os.path.join
, guiding you through its syntax, usage, and best practices. Whether you’re a beginner or an experienced coder, understanding os.path.join
is crucial for handling file paths in Python.
Key Takeaways:
Understanding os.path.join
Python’s os.path.join
method intelligently combines one or more path components. By ensuring that exactly one directory separator is placed between each non-empty part, it simplifies file path construction, making it more robust and less prone to errors.
Basics of File Path Management in Python
In Python, managing file paths is a common task, especially in applications involving file I/O operations. Hard-coding file paths can lead to issues, particularly when dealing with cross-platform compatibility. Here, os.path.join
comes into play, providing a way to construct file paths dynamically and correctly, regardless of the operating system.
Syntax and Parameters of os.path.join
The signature of os.path.join
is straightforward. It takes multiple path components as arguments and returns a single path string. Each argument is a string representing a path component, and the method concatenates these components using the appropriate file separator for the operating system.
import os
path = os.path.join('directory', 'subdirectory', 'file.txt')
In this example, os.path.join
creates a path by intelligently placing a directory separator (/
or \
depending on the OS) between ‘directory’, ‘subdirectory’, and ‘file.txt’.
Related Reading: Python: Convert List to a String | TraceDynamics
Common Use Cases of os.path.join
os.path.join
is versatile, applicable in various scenarios:
Use Case |
Description |
Basic File Path Construction |
Combining directory names and a file name to form a complete path. |
Dynamic Path Construction |
Building file paths in a loop or based on user input. |
Cross-platform Compatibility |
Creating paths that work on different operating systems. |
Handling Cross-Platform Path Differences
One of the most significant advantages of os.path.join
is its ability to handle differences in file path syntax across operating systems. For instance, Windows uses backslashes (\
), while UNIX-based systems use forward slashes (/
). os.path.join
automatically handles these differences.
Learn More: Python 3.12.0 os.path Documentation
Best Practices for Using os.path.join
To get the most out of os.path.join
, consider the following best practices:
- Avoid Hard-coding Separators: Let
os.path.join
handle the insertion of directory separators.
- Use with Other os.path Functions: Combine
os.path.join
with functions like os.path.abspath
or os.path.dirname
for more functionality.
- Error Checking: Always validate the inputs to
os.path.join
to avoid unexpected results.
Advanced Techniques Using os.path.join
While os.path.join
is primarily used for concatenating path components, its utility extends to more complex scenarios:
- Handling Variable Number of Path Components: You can use
os.path.join
with list comprehension or unpacking operators to dynamically create paths from a list of components.
- Integration with Environment Variables: Combine environment variables with
os.path.join
to construct paths relative to user-specific directories, like the home directory.
Related Reading: Git Merge Master into Branch: Advanced Merging Techniques
Integration with Other Python Modules
os.path.join
can be seamlessly integrated with other modules for enhanced functionality:
Error Handling and Debugging Tips
Effective error handling is crucial. Ensure the components passed to os.path.join
are strings. Be mindful of edge cases, like empty strings or special characters, and test your paths thoroughly.
Practical Examples and Case Studies
Case Study 1: Automating File Path Generation in Data Analysis
- Scenario: A data analyst needs to generate file paths for various datasets stored in different directories.
- Solution: Using
os.path.join
to dynamically create file paths based on dataset names and directory structures.
Case Study 2: Cross-Platform File Management in a Python Application
- Scenario: A Python application needs to work seamlessly on Windows and UNIX-based systems.
- Solution: Implementing
os.path.join
for all file path operations to ensure compatibility across platforms.
Frequently Asked Questions (FAQs)
What are the best practices for using `os.path.join` in Python?
Use `os.path.join` to ensure cross-platform compatibility, avoid hard-coding path separators, and combine it with other `os.path` functions for robust path manipulation.
Can `os.path.join` handle paths with special characters?
Yes, but ensure proper encoding and be cautious with characters that might be interpreted differently by the file system.
Is `os.path.join` necessary for simple file path operations?
While not always necessary, it’s recommended for cleaner, more maintainable code and to avoid platform-specific issues.
How does `os.path.join` work with absolute and relative paths?
`os.path.join` intelligently handles both absolute and relative paths, making it versatile for various applications.
What are common errors to avoid with `os.path.join`?
Avoid passing non-string arguments and be wary of edge cases, like empty strings or paths with unintended special characters.
Python
Python HashMap: In the realm of Python programming, hashmaps stand as a cornerstone of efficient data management, leveraging the power of the dictionary data structure to offer unparalleled speed and flexibility in handling key-value pairs. This comprehensive guide delves into the intricacies of Python hashmaps, exploring their implementation, advantages, and practical applications in real-world scenarios.
Key Takeaways:
Understanding Python HashMaps
What is a Python HashMap?
A hashmap in Python, implemented as a dictionary, is an indexed data structure that associates keys with values. The keys are unique and immutable, allowing for efficient data retrieval and management. In essence, a hashmap is akin to a labeled cabinet, where each drawer (bucket) is indexed for quick access.
- Python’s Dictionary as a HashMap:
- In Python, dictionaries (
dict
) are the native implementation of hashmaps.
- These are mutable, unordered collections of key-value pairs.
- Python dictionaries provide fast lookup, insertion, and deletion operations.
Example of a Python Dictionary:
my_dict = {'apple': 5, 'banana': 8, 'cherry': 3}
print(my_dict['banana']) # Output: 8
The Importance of Hash Functions
- Role in HashMaps: Hash functions convert keys into hash codes, which are then mapped to indexes in an array of buckets.
- Efficiency: A good hash function minimizes collisions and evenly distributes keys across buckets, enhancing lookup speed.
Table: Characteristics of an Effective Hash Function
Trait |
Description |
Deterministic |
Same input always yields the same hash code. |
Uniform Distribution |
Hash codes are spread evenly across the array. |
Fast Computation |
The function is quick to compute. |
Minimizes Collisions |
Fewer instances where different keys yield the same hash code. |
Advantages of Using HashMaps in Python
- Speed: Facilitates rapid data retrieval and manipulation.
- Flexibility: Accommodates a wide range of data types as keys and values.
- Dynamic Sizing: Automatically resizes to accommodate more entries.
Python HashMap Use Case:
- Inventory System: Efficiently track and update item quantities.
- User Database: Rapidly access user details using unique identifiers.
Implementing HashMaps in Python
Creating and Using a Python HashMap
Python Dictionary Operations Example:
# Initializing a dictionary
inventory = {'apples': 30, 'oranges': 20}
# Adding an element
inventory['bananas'] = 15
# Accessing an element
print(inventory['apples']) # Output: 30
Handling Collisions in Hashmaps
- Collision: Occurs when two keys hash to the same index.
- Resolution Strategies: Separate chaining, open addressing, etc.
- Python’s Approach: Python dictionaries handle collisions internally, ensuring consistent performance.
Table: Collision Resolution Techniques
Technique |
Description |
Separate Chaining |
Stores collided elements in a linked list at the index. |
Open Addressing |
Finds another index within the array using probing. |
Internal Workings of a HashMap
- Hash Function: Transforms keys into array indexes.
- Buckets and Slots: The array structure where values are stored.
- Collision Handling: Ensures unique mapping for each key.
Diagram: Structure of a Python HashMap
- A visual representation of keys being hashed to indexes and stored in an array.
HashMaps vs. Other Data Structures
Comparison with Lists and Sets
- Lists: Ordered collections, slower for search operations.
- Sets: Unordered collections of unique elements, faster than lists but do not store key-value pairs.
- HashMaps: Offer the best of both worlds with fast lookup times and key-value pair storage.
Table: Python Data Structures Comparison
Data Structure |
Ordered |
Unique Keys |
Key-Value Pairs |
Average Lookup Time |
List |
Yes |
No |
No |
O(n) |
Set |
No |
Yes |
No |
O(1) |
Dictionary (HashMap) |
No |
Yes |
Yes |
O(1) |
Performance and Limitations
Efficiency of Python HashMaps
- Time Complexity: O(1) average time complexity for lookup, insert, and delete operations.
- Space Complexity: Efficient storage, but memory usage increases with the number of entries.
Limitations and Considerations
- Memory Overhead: Larger than lists or tuples for a small number of elements.
- Mutable Keys: Keys must be immutable and hashable.
Python HashMap Performance Example:
- Fast Lookup: Retrieving user details from a large database.
Real-World Applications of HashMaps
Use Cases in Software Development
- Database Indexing: Enhancing retrieval speed.
- Caching Mechanisms: Storing temporary data for quick access.
- Algorithm Optimization: Reducing time complexity in algorithms.
Python HashMap Application Example:
- Web Caching System: Store web page content against URLs for quick retrieval.
Table: Real-World Applications of Python HashMaps
Application |
Description |
Web Caching |
Storing web pages for faster loading. |
Inventory Management |
Tracking item quantities in a store. |
User Authentication |
Quick lookup of user credentials. |
Advanced HashMap Operations in Python
Customizing Hash Functions
- Custom Hashing: Python allows the creation of custom hash functions for user-defined objects.
- Enhancing Performance: Tailoring hash functions to specific data distributions can optimize performance.
Example of Custom Hash Function:
class Product:
def __init__(self, id, name):
self.id = id
self.name = name
def __hash__(self):
return hash((self.id, self.name))
product_dict = {Product(1, 'Phone'): 500, Product(2, 'Laptop'): 1000}
Sorting and Iterating through HashMaps
- Order Maintenance: As of Python 3.7, dictionaries maintain insertion order.
- Sorting Techniques: Using lambda functions and itemgetter for custom sorting.
Python HashMap Sorting Example:
import operator
inventory = {'apples': 30, 'oranges': 20, 'bananas': 15}
sorted_inventory = sorted(inventory.items(), key=operator.itemgetter(1))
print(sorted_inventory) # Output: [('bananas', 15), ('oranges', 20), ('apples', 30)]
Scaling HashMaps for Large Datasets
- Handling Large Data: Python’s efficient memory management allows hashmaps to scale with large datasets.
- Optimization Strategies: Techniques like resizing and load factor adjustment.
Table: Scaling Strategies for Large HashMaps
Strategy |
Description |
Dynamic Resizing |
Automatically increases size when load factor exceeds a threshold. |
Load Factor Adjustment |
Modifying the load factor to balance between time and space efficiency. |
HashMaps in Data Analysis and Machine Learning
Data Structuring and Analysis
- Organizing Data: HashMaps offer a structured way to store and analyze complex datasets.
- Efficient Data Retrieval: Crucial for large-scale data processing tasks.
Machine Learning Applications
Python HashMap ML Example:
- Feature Lookup: Rapidly accessing features of a dataset for a machine learning algorithm.
Practical Insights and Performance Tuning
Monitoring and Improving HashMap Performance
- Profiling Tools: Utilizing Python profiling tools to monitor hashmap performance.
- Tuning Techniques: Adjusting hashmap parameters for optimal performance.
Best Practices for HashMap Usage
- Key Selection: Choosing appropriate keys for efficient hashing.
- Memory Management: Balancing between memory usage and performance.
Python HashMap Best Practices Example:
Conclusion
In conclusion, Python hashmaps, as a versatile and powerful data structure, offer a wide range of applications and benefits in various fields of software development, data analysis, and machine learning. By understanding their workings, advantages, and best practices, developers can effectively leverage hashmaps to optimize their Python applications.
Frequently Asked Questions (FAQs)
What makes Python hashmaps efficient for data retrieval?
Python hashmaps, implemented as dictionaries, offer O(1) average time complexity for lookup, making them extremely efficient for data retrieval.
Can Python hashmaps maintain the order of elements?
Yes, as of Python 3.7, dictionaries maintain the insertion order of elements.
How do Python hashmaps handle collisions?
Python hashmaps handle collisions internally using techniques like separate chaining and open addressing.
Are there limitations to using hashmaps in Python?
While highly efficient, Python hashmaps have a memory overhead and require immutable, hashable keys.
Can we use custom objects as keys in Python hashmaps?
Yes, custom objects can be used as keys in Python hashmaps by defining a custom hash function for them.
Python
Print Exception Python: In any programming environment, errors are inevitable ghosts in the code that developers must chase. Python, like other languages, has a robust system for handling them, making it easier to deal with the myriad of errors that could occur. This python tutorial dives into the core concepts of Python exceptions, explores ways to handle them effectively, and equips you with the tools to build reliable and resilient programs.
Key Takeaways:
Understanding Python Exceptions
Introduction to Python Exceptions
Imagine a scenario where you expect a file to be present, but it’s missing. This unexpected event triggers a FileNotFoundError
, notifying you of the discrepancy. Exceptions act as real-world standard error analogies in your code, allowing you to handle them gracefully and prevent python program crashes.
Defining the Terms:
- Error: A problem in the code that halts program execution.
- Exception: A special type of error that can be caught and handled.
- Traceback: A traceback module is a detailed record of the sequence of function calls that led to an exception, providing valuable insights for debugging.
Built-in Exception Classes:
All exceptions in Python belong to classes derived from the base Exception class. This hierarchy facilitates structured handling of different groups of exceptions.
Here’s a glimpse of the hierarchy:
- Base Class: Exception
- Derived Classes: ArithmeticError, LookupError, EnvironmentError, etc.
Commonly Used Built-in Classes:
- OSError: Base class for I/O-related errors.
- IndexError: Raised when a sequence subscript is out of range.
- ZeroDivisionError: Raised when attempting to divide by zero.
Real-world Analogy
try:
with open('file.txt', 'r') as file:
content = file.read()
except FileNotFoundError as e:
print(f"Error: {e}")
In the above code snippet, if file.txt
doesn’t exist, Python will throw a FileNotFoundError
. However, due to the try-except
block, this error will be caught and a user-friendly message will be printed instead of halting the program.
Common Types of Exceptions in Python
This infographic summarizes common exception types in Python.
Python has a number of built-in exceptions that are raised when your program encounters an error (something in the program goes awry). Here are some common mutiple exception you might encounter:
SyntaxError
: Raised when there is an error in Python syntax.
TypeError
: Raised when an operation or function is applied to an object of inappropriate type.
ValueError
: Raised when a built-in operation or function receives an argument that has the right type but an inappropriate value.
Unraveling Specific Exceptions: A Closer Look
While Python’s built-in exceptions cover a wide range of errors, let’s dive deeper into a few common ones to uncover their causes, consequences, and best practices for handling them gracefully:
KeyError: When Dictionaries Get Disgruntled
Imagine a dictionary as a meticulous librarian who demands precision when accessing its books (values). If you request a book by a title (key) that doesn’t exist, they’ll throw a KeyError, exclaiming, “That book isn’t in our collection!”
Common scenarios:
Searching for a non-existent key in a dictionary:
my_dict = {"name": "Alice", "age": 30}
try:
print(my_dict["occupation"]) # KeyError: 'occupation'
except KeyError as e:
print("Oops, missing key:", e)
Handling user input to access dictionary values:
user_choice = input("Choose a fruit: apple, banana, or orange: ")
try:
print("Your choice:", fruits[user_choice]) # Potential KeyError
except KeyError:
print("Invalid fruit choice. Please try again.")
Best practices:
Use get() for key retrieval with a default value:
occupation = my_dict.get("occupation", "Not specified")
Check for key existence with in before accessing:
if "occupation" in my_dict:
print(my_dict["occupation"])
IndexError: Out of Bounds with Lists and Tuples
Lists and tuples behave like organized shelves, each item indexed by a number. But venturing beyond their boundaries triggers an IndexError, a stern reminder that you’re trying to access an item that doesn’t exist.
Common scenarios:
Accessing elements beyond list or tuple length:
my_list = [10, 20, 30]
try:
print(my_list[3]) # IndexError: list index out of range
except IndexError:
print("Index out of bounds!")
Using negative indices incorrectly:
my_tuple = (5, 10)
try:
print(my_tuple[-3]) # IndexError: tuple index out of range
except IndexError:
print("Invalid negative index.")
Best practices:
- Double-check list or tuple lengths before indexing.
- Use positive indices starting from 0.
- Be mindful of negative indices, which count from the end.
Stay tuned as we delve into more intriguing exception types, unraveling their mysteries and unlocking best practices for handling them like a Python pro!
The Try-Except Block
block forms the bedrock of exception handling in Python. The try clause block houses code that might raise an specific exception, while the except
block defines how to handle it.
try:
# code that may raise exception
num = int("string")
except ValueError as ve:
print(f"Error: {ve}")
In the code above, a ValueError
will be raised because you cannot convert a string to an integer. The try-except
block catches the error and prints a user-friendly message.
Syntax and Usage
- The
try
block: Contains code that may raise an exception.
- The
except
block: Contains code that will execute if an exception occurs.
Example Scenarios
- Reading a file that may not exist.
- User input validation.
The below flowchart illustrates the try, catch and finally clause:
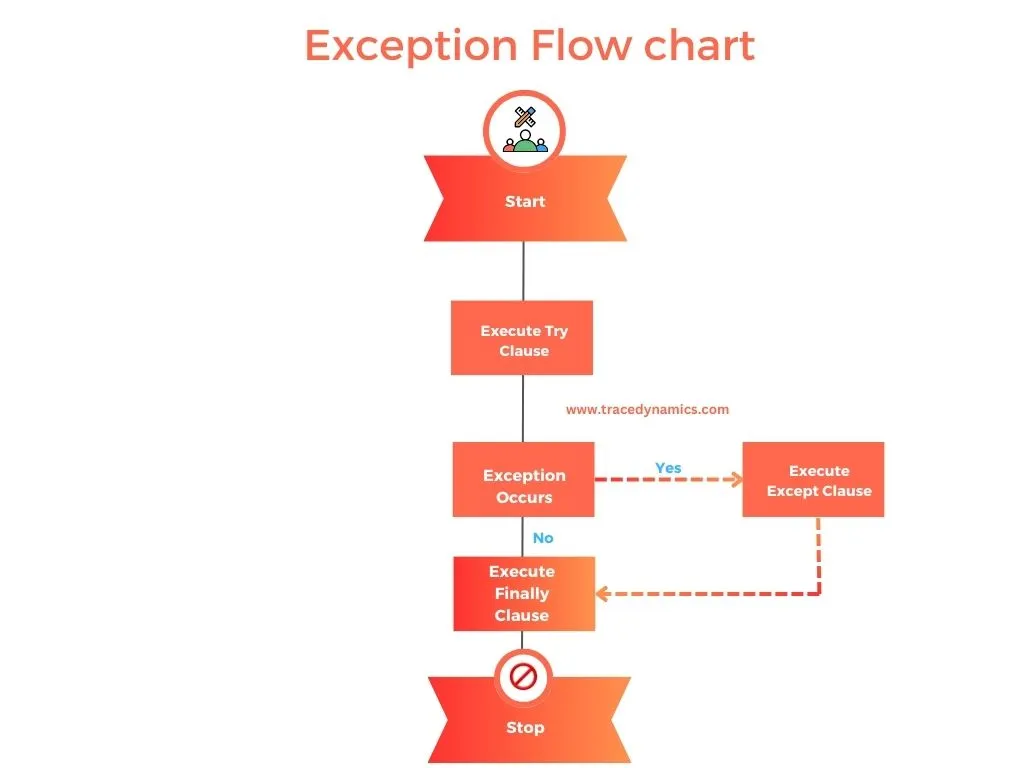
Handling Exceptions in Python
Handling or raising exceptions effectively is crucial for building robust applications. In Python, exception handling encompasses a broad spectrum from printing exception to logging them for post-mortem analysis. This section delves into various techniques to handle exceptions in Python
Printing Exceptions
Printing exceptions is a fundamental technique that provides valuable insights into the error without crashing the program. You can use the print
function to display the exception message and type for better understanding.
Using the print
Function to Display Exceptions
try:
# code that may raise exception
num = int("string")
except ValueError as ve:
print(f"Error: {ve}")
In this code, if a ValueError
occurs, its message and type will be printed, providing helpful information for debugging.
Formatting Exceptions for Better Readability
Python provides various ways to format exceptions, making it easier to understand the error.
try:
# code that may raise exception
num = int("string")
except ValueError as ve:
print(f"Error: {ve}")
print(f"Exception Type: {type(ve)}")
print(f"Traceback: {traceback.print_exc()}") # Prints the traceback
In this code, if a ValueError occurs, the message, type, and traceback will be printed, providing a comprehensive understanding of the error’s context.
Understanding the Power of the Python Traceback:
While we’ve been discussing how to catch and handle exceptions in Python, there’s another crucial tool in our debugging arsenal: the traceback. Think of it as a detective’s roadmap, leading you step-by-step through the code that led to the error. Understanding and analyzing tracebacks effectively can dramatically improve your debugging skills and help you pinpoint the culprit quickly.
What is a Traceback?
Imagine you’re making a delicious casserole, but it ends up tasting slightly off. Tracing back your steps helps you identify the ingredient or step that went wrong. Similarly, a traceback is a record of the function calls leading up to the error, offering a detailed chronological narrative of what went wrong.
Here’s an example:
def calculate_discount(price, discount_rate):
try:
final_price = price * (1 - discount_rate)
return final_price
except TypeError:
print("Error: Invalid input types!")
original_price = "100"
discount = 0.5
try:
final_price = calculate_discount(original_price, discount)
print(f"Final price: ${final_price}")
except TypeError:
print("Please enter valid number values.")
Running this code would result in a TypeError because “100” is a string, not a number. The traceback would look something like this:
Traceback (most recent call last):
File "recipe.py", line 6, in
final_price = calculate_discount(original_price, discount)
File "recipe.py", line 2, in calculate_discount
final_price = price * (1 - discount_rate)
TypeError: unsupported operand type(s) for *: 'str' and 'float'
This traceback tells us:
- The error occurred on line 2 of calculate_discount.
- It’s a TypeError because of an unsupported operation between a string and a float.
- The call originated from line 6 in the main block.
Decoding the Traceback:
Each line in the traceback represents a function call, with the most recent call at the bottom. Reading from the bottom up, you can trace the path the program took before encountering the error.
Analyzing the elements helps you pinpoint the issue:
- Filename and line number: Identify the exact location of the error.
- Function names: Understand the sequence of function calls leading to the error.
- Error message: Get an immediate clue about the nature of the issue.
Think of the traceback as a series of breadcrumbs leading you directly to the source of the problem. Analyzing each step systematically will help you fix the bug and prevent similar issues in the future.
Mastering Traceback Analysis:
Here are some additional tips for effectively using tracebacks:
- Shorten tracebacks: Use the traceback.format_exc() function to shorten long tracebacks for easier reading.
- Visualize the stack: Utilize tools like debuggers or online visualizers to create interactive stack diagrams for clearer understanding.
- Leverage additional resources: Consult online documentation and tutorials to learn about advanced traceback analysis techniques.
By incorporating these practices, you can transform from a passive traceback observer to a skilled code detective, confidently navigating the error landscape and ultimately delivering robust and well-functioning Python applications.
Remember: The next time you encounter an error in your Python code, don’t be intimidated by the traceback. Embrace it as a valuable tool to uncover the problem and write flawless code!
User-Friendly Exceptions: Turning Cryptic Errors into Helpful Guides
Errors are inevitable, but cryptic technical jargon shouldn’t be the norm. In Python, user-friendly exception printing transforms confusing messages into valuable tools that guide users towards solutions. Let’s explore best practices for making your exceptions helpful and informative:
- Ditch the Jargon: Translate technical terms like “ValueError” or “IndexError” into plain English. Think of explaining the error to a friend unfamiliar with coding. For example, instead of “TypeError: unsupported operand type(s) for +: ‘str’ and ‘int’,” say, “Oops! Adding text and numbers isn’t allowed here. Please use only numbers for this calculation.”
- Context is Key: Don’t leave users lost in the code. Provide context around the error by mentioning the specific function or line where it occurred. This saves them time hunting for the culprit. For example, “An error occurred while calculating your discount in the checkout function. Please double-check your discount code.”
- Offer a Helping Hand: Don’t just point out the problem; offer solutions! Suggest what the user can do to fix the error. For example, “Looks like you entered an invalid date format. Please use YYYY-MM-DD format for the date field.”
- Prioritize Like a Pro: Not all errors are created equal. Use different logging levels to distinguish minor hiccups from major roadblocks. A missing comma shouldn’t trigger panic, while a server crash deserves a bold “ERROR” flag.
- Format for Clarity: A wall of text is nobody’s friend. Use formatting like bullet points, indentation, or even colored text to make your error messages easy to scan and understand.
- Think Globally, Act Locally: Is your application reaching a global audience? Consider localization! Translate your error messages into different languages to ensure all users receive clear guidance regardless of their native tongue.
- Learn from the Best: Utilize libraries like prettyprinter or colorama to leverage their expertise in formatting and coloring error messages for optimal user experience.
- Less is More: While context and suggestions are valuable, avoid overwhelming users. Keep your messages concise and actionable. Remember, clarity and brevity are key to effective communication.
- Test and Iterate: Don’t assume your first draft is perfect. Test your user-friendly error messages with real users and gather their feedback. Use their insights to refine your messages for maximum impact.
- Remember the Mission: The ultimate goal is to empower users to navigate errors without technical expertise. By following these tips and prioritizing user-friendliness, you can turn frustrating roadblocks into manageable bumps on their code journey.
Logging Exceptions
Logging exceptions is a step beyond merely printing them. It records exceptions in a log file, aiding in debugging post-mortem.
Introduction to Logging in Python
Python’s logging module provides a flexible framework for emitting log messages from Python programs. It’s part of the Python Standard Library, so you don’t need to install anything to use it.
import logging
try:
# code that may raise exception
num = int("string")
except ValueError as ve:
logging.exception("Error")
In this code snippet, the logging.exception
method logs the exception with a message “Error”.
How to Log Exceptions
Logging exceptions involve creating a logging configuration and using logging methods to record exceptions.
import logging
logging.basicConfig(filename='example.log', level=logging.ERROR)
try:
# code that may raise exception
num = int("string")
except ValueError as ve:
logging.exception("Error")
In this code snippet, the logging.basicConfig
method is used to configure the logging, and logging.exception
is used to log the exception.
Custom Exception Classes
Creating user-defined exceptions allows for creating self-explanatory error messages and handling exceptions at a granular level.
Creating User-Defined Exceptions
class CustomError(Exception):
pass
try:
raise CustomError("A custom error occurred")
except CustomError as ce:
print(ce)
In this code snippet, a custom exception class CustomError
is created, which is derived from the base Exception
class.
Use Cases for Custom Exceptions
Custom exceptions can be used to create program-specific error conditions. They can also be used to create a hierarchy of exception classes for a large codebase.
Advanced Techniques:
Advanced exception handling involves techniques that provide more control over the exception handling/error handling process.
- Logging: Record exception name in a log file for post-mortem analysis.
- Custom Exceptions: Create custom exception classes for program-specific error conditions.
- Exception Chaining: Handle chained exceptions when one exception occurs while handling another.
- Context Managers: Simplify resource management and exception handling using
with
statements.
Exception Chaining
Exception chaining is used when an exception occurs while handling another exception.
try:
# code that may raise exception
num = int("string")
except ValueError as ve:
raise TypeError("A type error occurred") from ve
Context Managers for Exception Handling
Context managers simplify common resource management patterns by encapsulating standard uses of try statement/except/finally in so-called context management protocols.
with open('file.txt', 'r') as file:
content = file.read()
Remember:
Exception handling is crucial for building robust and reliable Python applications. Embrace these techniques to confidently confront errors and write bug-free python code!
Frequently Asked Questions (FAQs)
What is an exception in Python?
An exception in Python is an error that occurs during the execution of a program, which disrupts the normal flow of the program’s instructions.
How do I catch exceptions in Python?
In Python, exceptions can be caught using a try-except block. Code that may raise an exception is placed within the try block, and code that will execute in case of an exception is placed within the except block.
How do I raise exceptions in Python?
Exceptions can be raised using the raise statement followed by the name of the exception, and optionally followed by an error message.
Can I create custom exceptions in Python?
Yes, you can create custom exceptions in Python by creating a new class derived from the base Exception class.
What is exception chaining in Python?
Exception chaining occurs when an exception is raised in response to catching a different exception. This is useful for capturing and retaining the traceback information of the original exception.
How do I log exceptions in Python?
You can log exceptions in Python using the logging module. By configuring a logger, you can capture exception information and write it to a log file or other output streams.
What are some common built-in exceptions in Python?
Some common built-in exceptions in Python include ValueError, TypeError, IndexError, KeyError, and FileNotFoundError.
How can I handle multiple exceptions in Python?
Multiple exceptions can be handled by using multiple except blocks with a try block or by specifying multiple exceptions in a single except block using a tuple.
What is the finally block in Python?
The finally block in Python is a block of code that will be executed no matter what, whether an exception is raised or not. It is often used for cleanup code.
What is the difference between errors and exceptions in Python?
Errors are issues in a program that the programmer does not expect to occur, while exceptions are conditions that a programmer anticipates and writes code to handle.
Python
Python String Builder: Python, as a flexible and powerful programming language, offers various methods for string building, a crucial aspect of many programming projects. This guide explores the alternative techniques for string building in Python, given the absence of a built-in StringBuilder
class. Understanding these methods is essential for efficient string handling and can significantly enhance your programming skills in Python.
Key Takeaways:
Introduction to Python String Building
In Python, unlike some other programming languages like Java and C#, there isn’t a built-in StringBuilder
class. However, string building is a common necessity in programming, especially when dealing with large amounts of text or data processing. This segment unravels the concept of string building in Python and underscores the importance of understanding alternative methods for handling strings efficiently in your projects.
Python’s flexibility shines through the various methods it offers for string building. Below are some of the commonly used techniques:
Using Join Method
The str.join()
method is a straightforward and efficient way to concatenate strings in Python. It’s particularly useful when you have a list of strings that need to be joined into a single string.
# Example:
string_list = ["Python", "is", "awesome"]
result_string = " ".join(string_list)
print(result_string) # Output: Python is awesome
This method is efficient and often preferred for its readability and speed, especially when dealing with a large number of strings.
Using String Concatenation
String concatenation using the +
operator is another common method for building strings in Python. However, it’s not as efficient as the str.join()
method, especially when concatenating a large number of strings.
# Example:
string1 = "Python"
string2 = " is"
string3 = " awesome"
result_string = string1 + string2 + string3
print(result_string) # Output: Python is awesome
Using Concatenation Assignment Operator
The concatenation assignment operator (+=
) is another way to concatenate strings, similar to the +
operator. However, each concatenation creates a new string, which may lead to increased time and memory consumption with a large number of strings.
# Example:
result_string = "Python"
result_string += " is"
result_string += " awesome"
print(result_string) # Output: Python is awesome
Using String IO Module
The io.StringIO
class provides a convenient interface for reading and writing strings as file objects. This method can be particularly useful for string building when dealing with file-like operations on strings.
import io
# Example:
string_builder = io.StringIO()
string_builder.write("Python")
string_builder.write(" is")
string_builder.write(" awesome")
result_string = string_builder.getvalue()
string_builder.close()
print(result_string) # Output: Python is awesome
This method provides a high level of control and is efficient for handling large strings.
Comparative Analysis of String Building Methods
Understanding the differences between these methods can aid in choosing the right technique for your project. The table below summarizes the key aspects of each method:
Method |
Efficiency |
Ease of Use |
Suitable for Large Strings |
str.join() Method |
High |
High |
Yes |
String Concatenation |
Medium |
High |
No |
Concatenation Assignment |
Low |
High |
No |
io.StringIO Class |
High |
Medium |
Yes |
Advanced String Building Techniques
Beyond the basic string building methods, Python offers advanced techniques and libraries for more complex string handling tasks. Exploring these methods can unlock new possibilities and optimize your string handling operations in Python.
In the realm of Python, beyond the basic string building methods, there are advanced techniques and libraries that can be employed to handle more complex string manipulation tasks. These advanced techniques can be especially beneficial when working on large-scale projects or when performance is a critical factor. Here are some examples:
Memory Views
Memory view is a safe way to expose the buffer protocol in Python. It allows you to access the internal data of an object that supports the buffer protocol without copying.
# Example:
byte_array = bytearray('hello', 'utf-8')
mem_view = memoryview(byte_array)
print(mem_view[0]) # Output: 104 (ASCII value of 'h')
The array Module
The array
module provides an array()
function which can be used to create arrays of unicode characters. This can be a more efficient way of building strings when compared to concatenation.
# Example:
import array
char_array = array.array('u', 'hello ')
char_array.fromstring('world')
result_string = char_array.tounicode()
print(result_string) # Output: hello world
The ctypes Module
The ctypes
module provides C compatible data types and allows calling functions in dynamic link libraries/shared libraries. It can be used to build strings in Python, especially when performance is a critical factor.
# Example:
import ctypes
# Create a mutable string
mutable_string = ctypes.create_string_buffer("hello world")
print(mutable_string.value) # Output: b'hello world'
String Preallocation
Preallocating memory for a string by creating a list of a known size can be a more efficient way of building strings when the final size of the output is known in advance.
# Example:
size = 5
string_builder = [''] * size
string_builder[0] = 'h'
string_builder[1] = 'e'
string_builder[2] = 'l'
string_builder[3] = 'l'
string_builder[4] = 'o'
result_string = ''.join(string_builder)
print(result_string) # Output: hello
Regular Expressions
Regular expressions provide a powerful way to handle strings. The re
module in Python provides a wide range of tools for working with regular expressions.
# Example:
import re
string = "hello world"
modified_string = re.sub(r'world', 'Python', string)
print(modified_string) # Output: hello Python
These advanced string building techniques offer a more sophisticated approach to string manipulation in Python, allowing for optimized performance and more complex operations. Employing these methods in your projects can help achieve better performance and meet specific project requirements.
Frequently Asked Questions (FAQs)
Is there a built-in StringBuilder class in Python?
No, Python does not have a built-in StringBuilder class. However, alternative methods such as the `str.join()` method and the `io.StringIO` class can be used for string building.
How can I efficiently construct strings from multiple fragments in Python?
The `str.join()` method and the `io.StringIO` class are two efficient ways to construct strings from multiple fragments in Python.
Which string building method is most efficient in Python?
The `str.join()` method is often considered the most efficient for concatenating a large number of strings, followed by the `io.StringIO` class.
Can I create my own string builder class in Python?
Yes, you can create your own string builder class in Python. This allows for a customized string building process suited to your specific needs.
Why is string immutability important in Python?
String immutability ensures that once a string is created, it cannot be altered. This feature enhances the security and simplicity of code in Python.
Python
XOR in Python: In the realm of programming, understanding logical operators is crucial for efficient problem-solving and code optimization. One such logical operator is the XOR (Exclusive OR) which has a wide range of applications in computing. This article delves into the essence of XOR in Python, shedding light on its implementation and real-world applications.
Key Takeaways
Introduction to XOR
XOR, short for Exclusive OR, is a logical operation that returns a boolean value of True if and only if exactly one of the operands is True. This unique behavior makes it a valuable tool in various computing scenarios.
Understanding XOR
/sleep-python
- Definition: XOR is a type of logical operator that compares two binary values and returns a new binary value based on the comparison.
- Symbol: The XOR operation is denoted by the caret symbol (^) in Python.
- Uniqueness: Unlike the AND and OR operators, XOR only returns True when exactly one of the operands is True.
Implementing XOR in Python
Python provides multiple methods to perform the XOR operation, each with its unique syntax and use-cases.
Using the ^ Operator
- Syntax:
result = value1 ^ value2
- Examples:
5 ^ 3
returns 6
because 0101
XOR 0011
equals 0110
in binary.
Using Logical Operators
Practical Applications of XOR in Python
XOR finds its applications across a spectrum of computing tasks, showcasing its versatility and importance.
Binary Operations
Cryptography
- Simple Encryption and Decryption: XOR can be used for creating simple encryption and decryption algorithms.
- Hash Functions: XOR is used in the construction of hash functions which are fundamental to cryptography.
Performance Considerations
When utilizing XOR in Python, considering the performance implications is essential to ensure efficient code execution.
- Execution Time: The choice of method for implementing XOR may affect the execution time of your code.
- Memory Usage: Similarly, memory usage can be impacted based on the method chosen for XOR implementation.
Execution Time Analysis
- Operator Efficiency: Analyzing the efficiency of different XOR implementation methods.
- Benchmarking: Comparing execution time across different methods to find the optimal approach.
Memory Usage Analysis
- Memory Footprint: Understanding the memory footprint associated with different XOR implementation methods.
- Optimization Techniques: Exploring techniques to minimize memory usage while maintaining code efficiency.
XOR in Advanced Computing Tasks
Diving deeper into the realm of XOR, its applications extend to more advanced computing tasks. The exclusive nature of XOR makes it a handy tool in various scenarios.
Error Detection and Correction
- Parity Checking: XOR is used to generate parity bits for error checking in data communication.
- Hamming Code: Utilizing XOR for creating and verifying Hamming codes for error correction.
Hash Functions
- Creating Unique Identifiers: XOR can be used in hash functions to generate unique identifiers for data.
- Ensuring Data Integrity: Hash functions with XOR ensure data integrity by creating a checksum.
Frequently Asked Questions (FAQs)
What is XOR used for in Python?
XOR is utilized for binary operations, cryptography, error detection and correction, among other uses.
How is XOR different from other logical operators?
Unlike AND and OR, XOR returns true only when one of the operands is true, making it exclusive.
How to implement XOR for more than two variables in Python?
XOR can be implemented for multiple variables by performing XOR operation pairwise.
Are there libraries in Python that can be used for XOR operations?
While Python’s built-in functionalities are sufficient, libraries like NumPy provide additional support for XOR operations.
What are the performance considerations when using XOR?
Execution time and memory usage are vital performance considerations when using XOR.
Python
String Indices Must be Integers: Python is a powerful, flexible programming language, but like any language, it has its own set of rules and conventions. One of these rules concerns how you can access individual characters in strings, and if you break this rule, you’ll see an error message: “string indices must be integers.” This message is Python’s way of telling you that you’ve made a mistake, but what exactly does it mean, and how can you fix it?
Key Takeaways
What Does The Error Mean?
The error message “string indices must be integers” is straightforward once you understand the terminology. In Python, strings are sequences of characters, and each character in a string has an index number representing its position in the string. These index numbers start at 0 for the first character, 1 for the second character, and so on. The error occurs when you try to use something other than an integer to index a string.
Here’s a simple example to illustrate this error:
greeting = "Hello, world!"
print(greeting[5.5])
In this code snippet, we’re trying to access a character in the string greeting
using a float (5.5
) as the index, which will trigger the error.
Common Causes of the Error
There are several common scenarios where this error might occur:
- Incorrect Index Type: As mentioned earlier, trying to access a string index with a non-integer value will trigger this error.
- Iterating Through Strings Improperly: If you’re iterating through a string and using the wrong variable as an index, you might encounter this error.
Here’s a brief table showing some common mistakes and how to avoid them:
Common Mistake |
Correction |
Using a float as an index |
Use an integer instead |
Using a string as an index |
Use an integer instead |
Misusing a variable in a loop |
Ensure loop variable is an integer |
How Python Handles Indices
In Python, indices are used to access specific elements in iterable objects like strings, lists, and tuples. The indices must be integers, and they represent positions of elements within the iterable.
Examples Demonstrating the Error
Understanding the error in a real-world context makes it easier to avoid in the future. Here are some examples where this error might occur:
- Accessing String Characters with Incorrect Indices:
word = "Python"
print(word["P"]) # This will cause an error
In this example, trying to access a character using a string instead of an integer as the index will trigger the error
.
- Incorrectly Iterating Through a String:
for index in "hello":
print(index[0]) # This will cause an error
In this loop, index
is a string, not an integer, so trying to use it as an index will trigger the error.
How To Fix The Error
Fixing the “string indices must be integers” error requires identifying and correcting the incorrect indexing. Here are some steps to fix the error:
- Identify the Incorrect Indexing:
- Check the line number mentioned in the error message to find where the error occurred.
- Look at the indices you’re using to access strings and ensure they are integers.
- Correct the Indexing:
- Replace any non-integer indices with integer indices.
- If you’re using variables as indices, make sure they contain integer values before using them to access strings.
Preventing the Error
Preventing the error from occurring in the first place is the best way to deal with it. Here are some tips:
- Use Integer Variables: Always use integer variables when indexing strings.
- Check Index Types: Before using a variable as an index, check its type to ensure it’s an integer.
Additional Resources
More on Fixing and Preventing the Error
Understanding the Importance of Correct Indexing
Correct indexing is crucial for many many operations in Python. Whether you are manipulating strings, lists, or other types of data, you need to use the correct indices to access and modify data accurately. Incorrect indexing can lead to errors, incorrect data, and other issues.
Tools and Libraries for Better Indexing
Various tools and libraries can help with indexing in Python. These tools can catch incorrect indexing and other common mistakes before they cause errors in your code
Enhancing Your Python Skills
Dealing with common errors like “string indices must be integers” is part of the learning process in Python. As you gain experience and learn from mistakes, you’ll become a more proficient programmer.
Here are some resources to further enhance your Python skills:
Additional Insights
Understanding and resolving the “string indices must be integers” error is a stepping stone to mastering data manipulation in Python. As you delve deeper into Python programming, you’ll encounter many such errors, and learning to resolve them promptly will significantly enhance your coding skills.
Frequently Asked Questions
1. Why does Python require integer indices for strings?
Python, like many programming languages, uses integer indices to track the position of elements within iterables such as strings. This system allows for efficient access and manipulation of data.
2. Can other types of numbers be used as indices?
No, only integers can be used as indices in Python. Attempting to use other types of numbers, like floats, will result in a TypeError.
3. How can I convert a non-integer index to an integer?
You can use the int()
function to convert a non-integer value to an integer. For example, int(5.5)
will return 5
.
4. What should I do if I encounter this error in my code?
First, identify the line of code where the error is occurring. Check the indices you are using to access strings and ensure they are integers. If they are not, you will need to correct them.
5. Are there tools that can help prevent this error?
Yes, tools like PyLint
or Pyright
can help catch type errors before runtime, helping to prevent this and other similar errors.